WSActivate (C Function)
int WSActivate(WSLINK link)
activates a WSTP connection, waiting for the program at the other end to respond.
Details
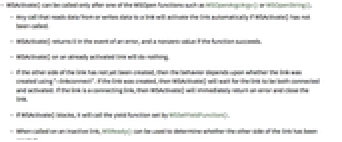
- WSActivate() can be called only after one of the WSOpen functions such as WSOpenArgcArgv() or WSOpenString().
- Any call that reads data from or writes data to a link will activate the link automatically if WSActivate() has not been called.
- WSActivate() returns 0 in the event of an error, and a nonzero value if the function succeeds.
- WSActivate() on an already activated link will do nothing.
- If the other side of the link has not yet been created, then the behavior depends upon whether the link was created using "-linkconnect". If the link was created, then WSActivate() will wait for the link to be both connected and activated. If the link is a connecting link, then WSActivate() will immediately return an error and close the link.
- If WSActivate() blocks, it will call the yield function set by WSSetYieldFunction().
- When called on an inactive link, WSReady() can be used to determine whether the other side of the link has been created.
- Use WSError() to retrieve the error code if WSActivate() fails.
- WSActivate() is declared in the WSTP header file wstp.h.
Examples
Basic Examples (1)
#include "wstp.h"
/* create a link and establish the connection */
int main(int argc, char **argv)
{
WSENV env;
WSLINK link;
int error;
env = WSInitialize((char *)0);
if(env == (WSENV)0)
{ /* unable to initialize the WSTP environment */ }
/* let WSOpenArgcArgv process the command line */
link = WSOpenArgcArgv(env, argc, argv, &error);
if(link == (WSLINK)0 || error != WSEOK)
{ /* unable to create the link */ }
/* WSActivate will establish the connection */
if(!WSActivate(link))
{ /* unable to establish communication */ }
/* ... */
WSClose(link);
WSDeinitialize(env);
return 0;
}