WSBytesToGet (C Function)
int WSBytesToGet(WSLINK link,int *n)
calculates the number of bytes left to read in the textual representation of the current data and stores the result in n.
Details
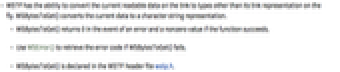
- WSTP has the ability to convert the current readable data on the link to types other than its link representation on the fly. WSBytesToGet() converts the current data to a character string representation.
- WSBytesToGet() returns 0 in the event of an error and a nonzero value if the function succeeds.
- Use WSError() to retrieve the error code if WSBytesToGet() fails.
- WSBytesToGet() is declared in the WSTP header file wstp.h.
Examples
Basic Examples (1)
#include <stdlib.h>
#include <string.h>
#include "wstp.h"
/* check the incoming string size to allocate enough memory to store the string */
void f(WSLINK lp)
{
int incoming_bytes;
char *string;
const char *wsstring;
switch(WSGetType(lp))
{
case WSTKSTR:
if(! WSBytesToGet(lp, &incoming_bytes))
{ /* unable to get the size of the string */ }
string = (char *)malloc(incoming_bytes + 1);
if(string == (char *)0)
{ /* memory allocation failed */ }
if(! WSGetString(lp, &wsstring))
{ /* unable to get the string from lp */ }
memcpy(string, wsstring, incoming_bytes);
*(string + incoming_bytes) = '\0';
break;
/* ... */
};
/* ... */
}