WSGetUnicodeString (C Function)
int WSGetUnicodeString(WSLINK link,unsigned short **s,long *n)
gets a character string from the WSTP connection specified by link, storing the string in s as a sequence of 16-bit Unicode characters.
Details
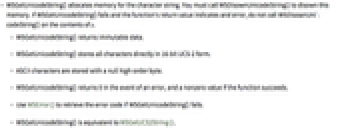
- WSGetUnicodeString() allocates memory for the character string. You must call WSDisownUnicodeString() to disown this memory. If WSGetUnicodeString() fails and the function's return value indicates and error, do not call WSDisownUnicodeString() on the contents of s.
- WSGetUnicodeString() returns immutable data.
- WSGetUnicodeString() stores all characters directly in 16-bit UCS-2 form.
- ASCII characters are stored with a null high-order byte.
- WSGetUnicodeString() returns 0 in the event of an error, and a nonzero value if the function succeeds.
- Use WSError() to retrieve the error code if WSGetUnicodeString() fails.
- WSGetUnicodeString() is equivalent to WSGetUCS2String().
Examples
Basic Examples (1)
#include "wstp.h"
/* read a UCS-2 encoded string from a link */
void f(WSLINK lp)
{
const unsigned short *string;
long length;
if(! WSGetUnicodeString(lp, &string, &length))
{
/* unable to read the UCS-2 string */
return;
}
/* ... */
WSDisownUnicodeString(lp, string, length);
}