WSPutNext (C Function)
int WSPutNext(WSLINK link,int type)
prepares to put an object of the specified type on link.
Details
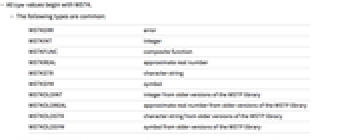
- All type values begin with WSTK.
- The following types are common:
-
WSTKERR error WSTKINT integer WSTKFUNC composite function WSTKREAL approximate real number WSTKSTR character string WSTKSYM symbol WSTKOLDINT integer from older versions of the WSTP library WSTKOLDREAL approximate real number from older versions of the WSTP library WSTKOLDSTR character string from older versions of the WSTP library WSTKOLDSYM symbol from older versions of the WSTP library - WSTKINT and WSTKREAL do not necessarily signify numbers that can be stored in C int and double variables.
- WSPutNext() returns 0 in the event of an error, and a nonzero value if the function succeeds.
- Use WSError() to retrieve the error code if WSPutNext() fails.
- WSPutNext() is declared in the WSTP header file mathlink.h.
Examples
Basic Examples (1)
#include "wstp.h"
/* send a function using tokens and argument counts to a link */
void f(WSLINK lp)
{
if(! WSPutNext(lp, WSTKFUNC))
{ /* unable to put the function type to lp */ }
if(! WSPutArgCount(lp, 2))
{ /* unable to put the number of arguments to lp */ }
if(! WSPutSymbol(lp, "Plus"))
{ /* unable to put the symbol to lp */ }
if(! WSPutInteger32(lp, 2))
{ /* unable to put the integer to lp */ }
if(! WSPutInteger32(lp, 3))
{ /* unable to put the integer to lp */ }
if(! WSFlush(lp))
{ /* unable to flush any buffered outgoing data to lp */ }
}