WSPutUTF16Function (C Function)
int WSPutUTF16Function(( WSLINK l , const unsigned short * s , int v , int n )
puts a function with head given by a UTF-16 encoded symbol with name s of length v and with n arguments to the WSTP connection specified by l.
Details
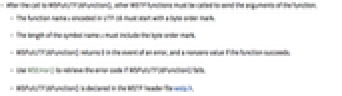
- After the call to WSPutUTF16Function(), other WSTP functions must be called to send the arguments of the function.
- The function name s encoded in UTF-16 must start with a byte order mark.
- The length of the symbol name s must include the byte order mark.
- WSPutUTF16Function() returns 0 in the event of an error, and a nonzero value if the function succeeds.
- Use WSError() to retrieve the error code if WSPutUTF16Function() fails.
- WSPutUTF16Function() is declared in the WSTP header file wstp.h.
Examples
Basic Examples (1)
#include "wstp.h"
/* A function to send List[1,2,3] to the link */
void f(WSLINK l)
{
unsigned short name[5];
name[0] = 0xFEFF;
name[1] = 'L';
name[2] = 'i';
name[3] = 's';
name[4] = 't';
if(! WSPutUTF16Function(l, (unsigned short *)name, 5, 3))
{ /* Unable to write the function head to the link */ }
if(! WSPutInteger8(l, 1))
{ /* Unable to write 1 to the link */ }
if(! WSPutInteger8(l, 2))
{ /* Unable to write 2 to the link */ }
if(! WSPutInteger8(l, 3))
{ /* Unable to write 3 to the link */ }
if(! WSEndPacket(l))
{ /* Unable to write the end-of-packet sequence to the link */ }
if(! WSFlush(l))
{ /* Unable to flush any outbound data from the link */ }
}