WSSetMessageHandler (C Function)
int WSSetMessageHandler(WSLINK link,WSMessageHandlerObject h)
installs the urgent message handler function referenced by h for for link.
Details
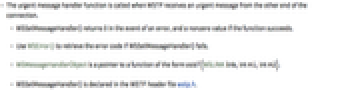
- The urgent message handler function is called when WSTP receives an urgent message from the other end of the connection.
- WSSetMessageHandler() returns 0 in the event of an error, and a nonzero value if the function succeeds.
- Use WSError() to retrieve the error code if WSSetMessageHandler() fails.
- WSMessageHandlerObject is a pointer to a function of the form void f(WSLINK link,int m1,int m2).
- WSSetMessageHandler() is declared in the WSTP header file wstp.h.
Examples
Basic Examples (1)
#include "wstp.h"
/* handle three common WSTP urgent messages */
void f(WSLINK lp, int msg, int arg)
{
if(msg == WSInterruptMessage)
{ /* generate an interrupt menu */ }
else if(msg == WSAbortMessage)
{ /* abort the current operation */ }
else if(msg == WSTerminateMessage)
{ /* shutdown the program */ }
/* ... */
}
int main(int argc, char **argv)
{
WSENV env;
WSLINK link;
int error;
env = WSInitialize((char *)0);
if(env == (WSENV)0)
{ /* unable to initialize WSTP environment */ }
link = WSOpenArgcArgv(env, argc, argv, &error);
if(link == (WSLINK)0 || error != WSEOK)
{ /* unable to create link */ }
if(! WSSetMessageHandler(link, (WSMessageHandlerObject)f)
{ /* unable to install message handler for link */ }
/* ... */
WSClose(link);
WSDeinitialize(env);
return 0;
}