WSUnicodeContainer (C Function)
is a WSTP type for storing UCS-2, UTF-8, UTF-16, or UTF-32 encoded strings and their lengths for easy passing of Unicode strings between functions in WSTP template programs.
Details
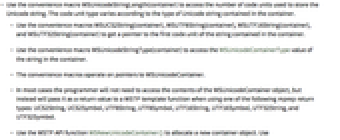
- Use the convenience macro WSUnicodeStringLength(container) to access the number of code units used to store the Unicode string. The code unit type varies according to the type of Unicode string contained in the container.
- Use the convenience macros WSUCS2String(container), WSUTF8String(container), WSUTF16String(container), and WSUTF32String(container) to get a pointer to the first code unit of the string contained in the container.
- Use the convenience macro WSUnicodeStringType(container) to access the WSUnicodeContainerType value of the string in the container.
- The convenience macros operate on pointers to WSUnicodeContainer.
- In most cases the programmer will not need to access the contents of the WSUnicodeContainer object, but instead will pass it as a return value to a WSTP template function when using one of the following mprep return types: UCS2String, UCS2Symbol, UTF8String, UTF8Symbol, UTF16String, UTF16Symbol, UTF32String, and UTF32Symbol.
- Use the WSTP API function WSNewUnicodeContainer() to allocate a new container object. Use WSReleaseUnicodeContainer() to release the memory used by the object.
- In most cases the programmer will not need to directly invoke WSReleaseUnicodeContainer() to free an WSUnicodeContainer object. Instead, mprep will generate code that automatically releases an WSUnicodeContainer object.
- WSUnicodeContainer is declared in the WSTP header file wstp.h.
Examples
Basic Examples (1)
#include "wstp.h"
/* A simple function for sending a Unicode string using a WSUnicodeContainer object */
void f(WSUnicodeContainer *container, WSLINK link)
{
if(container == (WSUnicodeContainer *)0)
return;
switch(WSUnicodeStringType(container))
{
case UCS2ContainerType:
if(! WSPutUCS2String(link, WSUCS2String(container),
WSUnicodeStringLength(container)))
{ /* Unable to send the UCS-2 encoded string */ }
break;
case UTF8ContainerType:
if(! WSPutUTF8String(link, WSUTF8String(container),
WSUnicodeStringLength(container)))
{ /* Unable to send the UTF-8 encoded string */ }
break;
case UTF16ContainerType:
if(! WSPutUTF16String(link, WSUTF16String(container),
WSUnicodeStringLength(container)))
{ /* Unable to send the UTF-16 encoded string */ }
break;
case UTF32ContainerType:
if(! WSPutUTF32String(link, WSUTF32String(container),
WSUnicodeStringLength(container)))
{ /* Unable to send the UTF-32 encoded string */ }
break;
}
WSReleaseUnicodeContainer(container);
}