WSYieldFunctionObject (C Function)
is a WSTP type that describes a function pointer to a function taking a WSLINK object as an argument and a WSYieldParameters object as an argument and returning an int.
Details
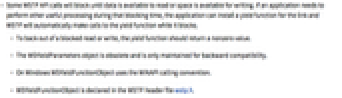
- Some WSTP API calls will block until data is available to read or space is available for writing. If an application needs to perform other useful processing during that blocking time, the application can install a yield function for the link and WSTP will automatically make calls to the yield function while it blocks.
- To back out of a blocked read or write, the yield function should return a nonzero value.
- The WSYieldParameters object is obsolete and is only maintained for backward compatibility.
- On Windows WSYieldFunctionObject uses the WINAPI calling convention.
- WSYieldFunctionObject is declared in the WSTP header file wstp.h.
Examples
Basic Examples (1)
#include "wstp.h"
#if UNIX_WSTP
# define CALLING_CONVENTION
#elif WINDOWS_WSTP
# define CALLING_CONVENTION WINAPI
#endif
int CALLING_CONVENTION AppYieldFunction(WSLINK lp, WSYieldParameters yp)
{
/* ... */
return 0;
}
int main(int argc, char **argv)
{
WSENV env;
WSLINK link;
int error;
env = WSInitialize((WSEnvironmentParameter)0);
if(env == (WSENV)0)
{ /* unable to initialize WSTP environment */ }
link = WSOpenArgcArgv(env, argv, argv + argc, &error);
if(link == (WSLINK)0 || error != WSEOK)
{ /* unable to create the link */ }
if(! WSSetYieldFunction(link, AppYieldFunction))
{ /* unable to set the yield function for link */ }
/* ... */
WSClose(link);
WSDeinitialize(env);
return 0;
}