MLGetNumberAsUTF32String (C Function)
MLGetNumberAsUTF32String has been replaced by WSGetNumberAsUTF32String.
int MLGetNumberAsUTF32String(MLINK l, const unsigned int **s, int n)
reads the next number on the MathLink connection specified by l as a string of UTF-32 characters representing the number value stored in the string s of length n.
Details
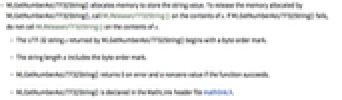
- MLGetNumberAsUTF32String() allocates memory to store the string value. To release the memory allocated by MLGetNumberAsUTF32String(), call MLReleaseUTF32String() on the contents of s. If MLGetNumberAsUTF32String() fails, do not call MLReleaseUTF32String() on the contents of s.
- The UTF-32 string s returned by MLGetNumberAsUTF32String() begins with a byte order mark.
- The string length n includes the byte order mark.
- MLGetNumberAsUTF32String() returns 0 on error and a nonzero value if the function succeeds.
- MLGetNumberAsUTF32String() is declared in the MathLink header file mathlink.h.
Examples
Basic Examples (1)
#include "mathlink.h"
/* A function for reading an integer from a link */
void f(MLINK l)
{
switch(MLGetType(l))
{
case MLTKINT:
{
int rawType;
rawType = MLGetRawType(l);
if(rawType == MLTK_MLSHORT)
{
short theNumber;
MLGetInteger16(l, &theNumber);
/* ... */
}
else if(rawType == MLTK_MLINT)
{
int theNumber;
MLGetInteger32(l, &theNumber);
/* ... */
}
else
{
const int *theNumber;
int length;
MLGetNumberAsUTF32String(l, &theNumber, &length);
/* ... */
MLReleaseUTF32String(l, theNumber, length);
}
}
break;
case MLTKREAL:
/* ... */
}
}