MLNewUnicodeContainer (C Function)
MLNewUnicodeContainer has been replaced by WSNewUnicodeContainer.
MLUnicodeContainer * MLNewUnicodeContainer(void *s, int l, enum MLUnicodeContainerType t)
allocates and returns a new Unicode container containing a copy of the contents of the Unicode string s of length l, a string of type t.
Details
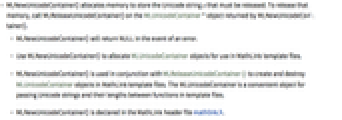
- MLNewUnicodeContainer() allocates memory to store the Unicode string s that must be released. To release that memory, call MLReleaseUnicodeContainer() on the MLUnicodeContainer * object returned by MLNewUnicodeContainer().
- MLNewUnicodeContainer() will return NULL in the event of an error.
- Use MLNewUnicodeContainer() to allocate MLUnicodeContainer objects for use in MathLink template files.
- MLNewUnicodeContainer() is used in conjunction with MLReleaseUnicodeContainer() to create and destroy MLUnicodeContainer objects in MathLink template files. The MLUnicodeContainer is a convenient object for passing Unicode strings and their lengths between functions in template files.
- MLNewUnicodeContainer() is declared in the MathLink header file mathlink.h.
Examples
Basic Examples (1)
#include "mathlink.h"
/* A MathLink template program for converting a string to a symbol */
:Begin:
:Function: convertStringToSymbol
:Pattern: ConvertStringToSymbol[string_String]
:Arguments: {string}
:ArgumentTypes: {String}
:ReturnType: UTF8Symbol
:End:
MLUnicodeContainer * convertStringToSymbol(const char *s)
{
MLUnicodeContainer *newContainer;
MLINK link;
int error;
unsigned char *utf8String;
int utf8Length, utf8Chars;
/* Use a loopback link to convert the string from Mathematica
form to a UTF-8 encoded version */
link = MLLoopbackOpen(stdenv, &error);
if(link == NULL || error != MLEOK)
{ /* Unable to create loopback link */ }
if(! MLPutString(link, s))
{ /* Unable to send the string to the loopback link */ }
if(! MLFlush(link))
[ /* Unable to flush the link buffers */ }
if(! MLGetUTF8String(link, &utf8String, &utf8Length, &utf8Chars))
{ /* Unable to read the UTF-8 encoded string */ }
/* The MLUnicodeContainer object is just for passing around
Unicode strings and their lengths in an easy manner. The
MathLink template code will call MLReleaseUnicodeContainer()
for the memory allocated by MLNewUnicodeContainer() */
/* UTF8ContainerType is the enum value that indicates the
MLUnicodeContainer object contains an UTF-8 encoded string */
newContainer = MLNewUnicodeContainer(utf8String, utf8Length,
UTF8ContainerType);
MLReleaseUTF8String(link, utf8String, utf8Length);
return newContainer;
}
int main(int argc, char **argv)
{
return MLMain(argc, argv);
}