MLUnicodeContainer (C Function)
MLUnicodeContainer has been replaced by WSUnicodeContainer.
is a MathLink type for storing UCS-2, UTF-8, UTF-16, or UTF-32 encoded strings and their lengths for easy passing of Unicode strings between functions in MathLink template programs.
Details
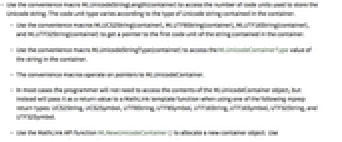
- Use the convenience macro MLUnicodeStringLength(container) to access the number of code units used to store the Unicode string. The code unit type varies according to the type of Unicode string contained in the container.
- Use the convenience macros MLUCS2String(container), MLUTF8String(container), MLUTF16String(container), and MLUTF32String(container) to get a pointer to the first code unit of the string contained in the container.
- Use the convenience macro MLUnicodeStringType(container) to access the MLUnicodeContainerType value of the string in the container.
- The convenience macros operate on pointers to MLUnicodeContainer.
- In most cases the programmer will not need to access the contents of the MLUnicodeContainer object, but instead will pass it as a return value to a MathLink template function when using one of the following mprep return types: UCS2String, UCS2Symbol, UTF8String, UTF8Symbol, UTF16String, UTF16Symbol, UTF32String, and UTF32Symbol.
- Use the MathLink API function MLNewUnicodeContainer() to allocate a new container object. Use MLReleaseUnicodeContainer() to release the memory used by the object.
- In most cases the programmer will not need to directly invoke MLReleaseUnicodeContainer() to free an MLUnicodeContainer object. Instead, mprep will generate code that automatically releases an MLUnicodeContainer object.
- MLUnicodeContainer is declared in the MathLink header file mathlink.h.
Examples
Basic Examples (1)
#include "mathlink.h"
/* A simple function for sending a Unicode string using a MLUnicodeContainer object */
void f(MLUnicodeContainer *container, MLINK link)
{
if(container == (MLUnicodeContainer *)0)
return;
switch(MLUnicodeStringType(container))
{
case UCS2ContainerType:
if(! MLPutUCS2String(link, MLUCS2String(container),
MLUnicodeStringLength(container)))
{ /* Unable to send the UCS-2 encoded string */ }
break;
case UTF8ContainerType:
if(! MLPutUTF8String(link, MLUTF8String(container),
MLUnicodeStringLength(container)))
{ /* Unable to send the UTF-8 encoded string */ }
break;
case UTF16ContainerType:
if(! MLPutUTF16String(link, MLUTF16String(container),
MLUnicodeStringLength(container)))
{ /* Unable to send the UTF-16 encoded string */ }
break;
case UTF32ContainerType:
if(! MLPutUTF32String(link, MLUTF32String(container),
MLUnicodeStringLength(container)))
{ /* Unable to send the UTF-32 encoded string */ }
break;
}
MLReleaseUnicodeContainer(container);
}