MLPutUTF32String (C Function)
MLPutUTF32String has been replaced by WSPutUTF32String.
int MLPutUTF32String(MLINK link,const unsigned int *s,int len)
puts a UTF-32 string s of length len to the MathLink connection specified by link.
Details
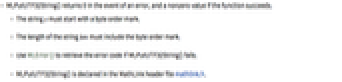
- MLPutUTF32String() returns 0 in the event of an error, and a nonzero value if the function succeeds.
- The string s must start with a byte order mark.
- The length of the string len must include the byte order mark.
- Use MLError() to retrieve the error code if MLPutUTF32String() fails.
- MLPutUTF32String() is declared in the MathLink header file mathlink.h.
Examples
Basic Examples (1)
#include "mathlink.h"
/* send the expression N[10/Sin[2]] as a UTF-32 string to a link */
void f(MLINK lp)
{
unsigned int expr[13];
expr[0] = 0xFEFF;
expr[1] = 'N';
expr[2] = '[';
expr[3] = '1';
expr[4] = '0';
expr[5] = '/';
expr[6] = 'S';
expr[7] = 'i';
expr[8] = 'n';
expr[9] = '[';
expr[10] = '2';
expr[11] = ']';
expr[12] = ']';
if(! MLPutFunction(lp, "EvaluatePacket", 1))
{ /* unable to put the function to lp */ }
if(! MLPutFunction(lp, "ToExpression", 1))
{ /* unable to put the function to lp */ }
if(! MLPutUTF32String(lp, (const unsigned int *)expr, 13))
{ /* unable to put the expression string to lp */ }
if(! MLEndPacket(lp))
{ /* unable to put the end-of-packet indicator to lp */ }
if(! MLFlush(lp))
{ /* unable to flush any outgoing data buffered in lp */ }
}