WSGetNumberAsUTF16String (C Function)
int WSGetNumberAsUTF16String(WSLINK l, const unsigned short **s, int *v, int *c)
reads the next number of the WSTP connection specified by l as a string of UTF-16 characters representing the number value stored in the string s of length v and characters c.
Details
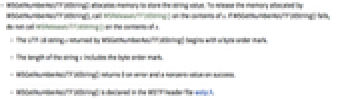
- WSGetNumberAsUTF16String() allocates memory to store the string value. To release the memory allocated by WSGetNumberAsUTF16String(), call WSReleaseUTF16String() on the contents of s. If WSGetNumberAsUTF16String() fails, do not call WSReleaseUTF16String() on the contents of s.
- The UTF-16 string s returned by WSGetNumberAsUTF16String() begins with a byte order mark.
- The length of the string v includes the byte order mark.
- WSGetNumberAsUTF16String() returns 0 on error and a nonzero value on success.
- WSGetNumberAsUTF16String() is declared in the WSTP header file wstp.h.
Examples
Basic Examples (1)
#include "wstp.h"
/* A function for reading an integer from a link */
void f(WSLINK l)
{
switch(WSGetType(l))
{
case WSTKINT:
{
int rawType;
rawType = WSGetRawType(l);
if(rawType == WSTK_WSSHORT)
{
short theNumber;
WSGetInteger16(l, &theNumber);
/* ... */
}
else if(rawType == WSTK_WSINT)
{
int theNumber;
WSGetInteger32(l, &theNumber);
/* ... */
}
else
{
const unsigned short *theNumber;
int length, characters;
WSGetNumberAsUTF16String(l, &theNumber, &length, &characters);
/* ... */
WSReleaseUTF16String(l, theNumber, length);
}
}
break;
case WSTKREAL:
/* ... */
}
}