WSGetNumberAsUTF32String (C Function)
int WSGetNumberAsUTF32String(WSLINK l, const unsigned int **s, int n)
reads the next number on the WSTP connection specified by l as a string of UTF-32 characters representing the number value stored in the string s of length n.
Details
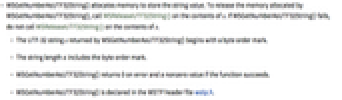
- WSGetNumberAsUTF32String() allocates memory to store the string value. To release the memory allocated by WSGetNumberAsUTF32String(), call WSReleaseUTF32String() on the contents of s. If WSGetNumberAsUTF32String() fails, do not call WSReleaseUTF32String() on the contents of s.
- The UTF-32 string s returned by WSGetNumberAsUTF32String() begins with a byte order mark.
- The string length n includes the byte order mark.
- WSGetNumberAsUTF32String() returns 0 on error and a nonzero value if the function succeeds.
- WSGetNumberAsUTF32String() is declared in the WSTP header file wstp.h.
Examples
Basic Examples (1)
#include "wstp.h"
/* A function for reading an integer from a link */
void f(WSLINK l)
{
switch(WSGetType(l))
{
case WSTKINT:
{
int rawType;
rawType = WSGetRawType(l);
if(rawType == WSTK_WSSHORT)
{
short theNumber;
WSGetInteger16(l, &theNumber);
/* ... */
}
else if(rawType == WSTK_WSINT)
{
int theNumber;
WSGetInteger32(l, &theNumber);
/* ... */
}
else
{
const int *theNumber;
int length;
WSGetNumberAsUTF32String(l, &theNumber, &length);
/* ... */
WSReleaseUTF32String(l, theNumber, length);
}
}
break;
case WSTKREAL:
/* ... */
}
}