WSRegisterCallbackFunction (C Function)
WSRegisterCallbackFunction
is a WSTP type that describes a function pointer to a function taking an WSENV object, an WSServiceRef object, an int object, a const char * object, and a void * object as arguments and returning void.
Details
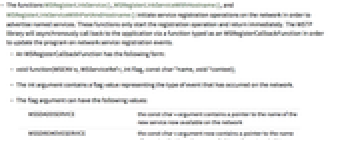
- The functions WSRegisterLinkService(), WSRegisterLinkServiceWithHostname(), and WSRegisterLinkServiceWithPortAndHostname() initiate service registration operations on the network in order to advertise named services. These functions only start the registration operation and return immediately. The WSTP library will asynchronously call back to the application via a function typed as an WSRegisterCallbackFunction in order to update the program on network service registration events.
- An WSRegisterCallbackFunction has the following form:
- void function( WSENV e, WSServiceRef r, int flag, const char *name, void *context);
- The int argument contains a flag value representing the type of event that has occurred on the network.
- The flag argument can have the following values:
-
WSSDADDSERVICE the const char * argument contains a pointer to the name of the new service now available on the network WSSDREMOVESERVICE the const char * argument now contains a pointer to the name of a service that is no longer available on the network; this function will only receive remove flags for services registered with the WSRegisterLinkService() functions that use this callback function
Examples
Basic Examples (1)
#include "wstp.h"
void RegisterCallbackFunction(WSENV e, WSServiceRef r, int flags, const char *serviceName, void *context);
WSServiceRef startRegisterOperation(WSENV e, const char *serviceName)
{
WSServiceRef theRef;
WSLINK theLink = (WSLINK)0;
int error;
theLink = WSRegisterLinkService(e,
serviceName,
RegisterCallbackFunction, NULL /* Use the default
network domain */, NULL, /* No context object for this
example */, &theRef, &error);
if(theLink == (WSLINK)0 || error != 0)
{ /* handle the error */ }
return theRef;
}
void RegisterCallbackFunction(WSENV e, WSServiceRef r, int flags, const char *serviceName, void *context)
{
if(flags & WSSDADDSERVICE)
{ /* Handle successful service registration */ }
else if(flags & WSSDREMOVESERVICE)
{ /* Handle service registration failure */ }
else if(flags & WSSDREGISTERERROR)
{ /* Handle error in register operation */ }
}