WSRegisterLinkService (C Function)
WSLINK WSRegisterLinkService(WSENV e, const char *p, const char *n, WSRegisterCallbackFunction f, const char *d, void *c, WSServiceRef *r, int *err)
starts the process of advertising the WSTP service named n, for the protocol p on the network, advertising the service on network domain d, asynchronously notifying the application of the registration results via the callback function f, and passing f the context object c and the resolve operation reference r.
Details
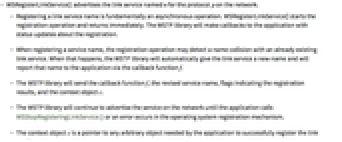
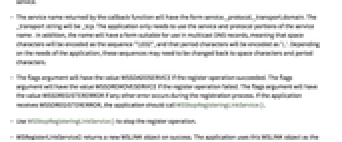
- WSRegisterLinkService() advertises the link service named n for the protocol p on the network.
- Registering a link service name is fundamentally an asynchronous operation. WSRegisterLinkService() starts the registration operation and returns immediately. The WSTP library will make callbacks to the application with status updates about the registration.
- When registering a service name, the registration operation may detect a name collision with an already existing link service. When that happens, the WSTP library will automatically give the link service a new name and will report that name to the application via the callback function f.
- The WSTP library will send the callback function f, the revised service name, flags indicating the registration results, and the context object c.
- The WSTP library will continue to advertise the service on the network until the application calls WSStopRegisteringLinkService() or an error occurs in the operating system registration mechanism.
- The context object c is a pointer to any arbitrary object needed by the application to successfully register the link service.
- The service name returned by the callback function will have the form service._protocol._transport.domain. The _transport string will be _tcp. The application only needs to use the service and protocol portions of the service name . In addition, the name will have a form suitable for use in multicast DNS records, meaning that space characters will be encoded as the sequence "\\032", and that period characters will be encoded as '\.'. Depending on the needs of the application, these sequences may need to be changed back to space characters and period characters.
- The flags argument will have the value WSSDADDSERVICE if the register operation succeeded. The flags argument will have the value WSSDREMOVESERVICE if the register operation failed. The flags argument will have the value WSSDREGISTERERROR if any other error occurs during the registration process. If the application receives WSSDREGISTERERROR, the application should call WSStopRegisteringLinkService().
- Use WSStopRegisteringLinkService() to stop the register operation.
- WSRegisterLinkService() returns a new WSLINK object on success. The application uses this WSLINK object as the listening end of the link service connection. The application must call WSActivate() on the link prior to using any other of the WSTP API functions.
- WSRegisterLinkService() will return NULL on failure and the err argument will contain an error code indicating the cause of the error. The error code corresponds to the WSE error codes in wstp.h and as returned by WSError().
Examples
Basic Examples (1)
#include "wstp.h"
void RegisterCallbackFunction(WSENV e, WSServiceRef r, int flags, const char *serviceName, void *context);
WSServiceRef startRegisterOperationForServiceWithProtocol(WSENV e,
const char *serviceName, const char *protocol)
{
WSServiceRef theRef;
WSLINK theLink = (WSLINK)0;
int error;
theLink = WSRegisterLinkService(e,
protocol, serviceName,
RegisterCallbackFunction, NULL /* Use the default
network domain */, NULL, /* No context object for this
example */, &theRef, &error);
if(theLink == (WSLINK)0 || error != 0)
{ /* handle the error */ }
return theRef;
}
void RegisterCallbackFunction(WSENV e, WSServiceRef r, int flags, const char *serviceName, void *context)
{
if(flags & WSSDADDSERVICE)
{ /* Handle successful service registration */ }
else if(flags & WSSDREMOVESERVICE)
{ /* Handle service registration failure */ }
else if(flags & WSSDREGISTERERROR)
{ /* Handle error in register operation */ }
}