WSResolveLinkService (C Function)
int WSResolveLinkService(WSENV e, WSResolveCallbackFunction f, const char *p, const char *n, void *c, WSServiceRef *r)
starts querying the network to resolve the connection details for the link service named n, a service for protocol p, asynchronously notifying the application of the connection details via the callback function f, passing f the context object c and the resolve operation reference r.
Details
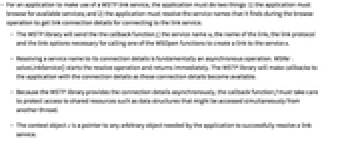
- For an application to make use of a WSTP link service, the application must do two things: 1) the application must browse for available services; and 2) the application must resolve the service names that it finds during the browse operation to get link connection details for connecting to the link service.
- The WSTP library will send the the callback function f, the service name n, the name of the link, the link protocol and the link options necessary for calling one of the WSOpen functions to create a link to the service n.
- Resolving a service name to its connection details is fundamentally an asynchronous operation. WSResolveLinkService() starts the resolve operation and returns immediately. The WSTP library will make callbacks to the application with the connection details as those connection details become available.
- Because the WSTP library provides the connection details asynchronously, the callback function f must take care to protect access to shared resources such as data structures that might be accessed simultaneously from another thread.
- The context object c is a pointer to any arbitrary object needed by the application to successfully resolve a link service.
- Use WSStopResolvingLinkService() to stop the resolve operation.
- WSResolveLinkService() returns 0 if the function succeeds and a nonzero value if the function fails.
- The nonzero values returned from WSResolveLinkService() as a result of an error correspond to the WSE error codes in wstp.h and as returned by WSError().
Examples
Basic Examples (1)
#include <string.h> /* for memset, memcpy, etc... */
#include <stdio.h> /* for snprintf */
#include "wstp.h"
void ResolveCallbackFunction(WSENV e, WSServiceRef r, const char *serviceName, const char *linkName, const char *protocol, int options, void *context);
WSServiceRef startResolvingServiceWithNameAndProtocol(WSENV e,
const char *name, const char *protocol)
{
WSServiceRef theRef;
int apiResult = 0;
apiResult = WSResolveLinkService(e, ResolveCallbackFunction,
protocol, name, NULL /* No context object for this example */,
&theRef);
if(apiResult != 0)
{ /* Handle the error */ }
return theRef;
}
void ResolveCallbackFunction(WSENV e, WSServiceRef r, const char *serviceName, const char *linkName, const char *protocol, int options, void *context)
{
WSLINK newLink = (WSLINK)0;
int error = 0;
int argc = 0;
const char *argv[] = {
"Dummy value representing program name",
"-linkname",
(const char *)0,
"-linkprotocol",
(const char *)0,
"-linkconnect",
"-linkoptions",
(const char *)0,
/* The following NULL pointer terminates the argv array */
(const char *)0
};
char optionsBuffer[50];
memset((void *)optionsBuffer, 0, sizeof(char) * 50));
snprintf(optionsBuffer, 50, "%d", options);
argv[2] = linkName;
argv[4] = protocol;
argv[7] = (const char *)optionsBuffer;
argc = 8;
newLink = WSOpenArgcArgv(e, argc, argv, &error);
if(newLink == (WSLINK)0 || error != WSEOK)
{ /* Handle the error */ }
/* ... */
return;
}