WSBrowseForLinkServices()
int WSBrowseForLinkServices(WSENV e,WSBrowseCallbackFunction f,const char *p,const char *d,void *c,WSServiceRef *r)
starts browsing the network for WSTP services matching protocol p, in network domain d, asynchronously notifying the application of service statuses via the callback function f passing f the context object c and the browse operation reference r.
Details
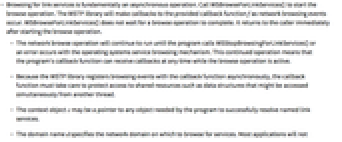
- Browsing for link services is fundamentally an asynchronous operation. Call WSBrowseForLinkServices() to start the browse operation. The WSTP library will make callbacks to the provided callback function f as network browsing events occur. WSBrowseForLinkServices() does not wait for a browse operation to complete. It returns to the caller immediately after starting the browse operation.
- The network browse operation will continue to run until the program calls WSStopBrowsingForLinkServices() or an error occurs with the operating systems service browsing mechanism. This continued operation means that the program's callback function can receive callbacks at any time while the browse operation is active.
- Because the WSTP library registers browsing events with the callback function asynchronously, the callback function must take care to protect access to shared resources such as data structures that might be accessed simultaneously from another thread.
- The context object c may be a pointer to any object needed by the program to successfully resolve named link services.
- The domain name d specifies the network domain on which to browse for services. Most applications will not specify a domain name and will instead pass NULL for d, indicating that the WSBrowseForLinkServices() should browse for services on the default domain.
- Use WSStopBrowsingForLinkServices() to stop the browse operation.
- The user must call WSBrowseForLinkServices() for each protocol p for which the user wants to receive browse event callbacks.
- WSBrowseForLinkServices() returns 0 if the function succeeds and a nonzero value if the function fails.
- The nonzero values returned from WSBrowseForLinkServices() as a result of an error correspond to the WSE error codes in wstp.h and as returned by WSError().
Examples
Basic Examples (1)
#include "wstp.h"
void BrowseCallbackFunction(WSENV e, WSServiceRef r, int flag, const char *serviceName, void *context);
void doWSTPServiceBrowsing(WSENV e, const char *protocol)
{
int apiResult;
WSServiceRef r;
apiResult = WSBrowseForLinkServices(env, BrowseCallbackFunction,
protocol, NULL /* For the default browse domain */, NULL, &r);
if(apiResult != 0)
{ /* handle error */}
/* Perform other tasks while browsing occurs */
/* For completeness stop the browse operation, this would normally
be handled at another point in the code */
WSStopBrowsingForLinkServices(e, r);
}
void BrowseCallbackFunction(WSENV e, WSServiceRef r, int flag, const char *serviceName, void *context)
{
if(flag & WSSDADDSERVICE)
{
/* The service named in serviceName has become available
on the network, store the name for use in the program. Don't
forget to include any synchronization mechanisms needed for
ensuring the integrity of shared data structures. */
}
else if(flag & WSSDREMOVESERVICE)
{
/* The service named in serviceName is no longer available
on the network, remove the name from any stored account of
named services on the network. Again, don't forget to make
use of synchronization mechanisms to ensure application integrity. */
}
else if(flag & WSSDBROWSEERROR)
{
/* An error has occurred with the browse operation. When this case happens, the program should use
WSStopBrowsingForLinkServices() to shutdown the browse
operation. An error communicating with the operating system
service discovery mechanisms has occurred and the browse
should be stopped and re-started. In addition, when
WSSDBROWSEERROR occurs, all cached service names should be
released. */
}
}