MLError (C Function)
MLError has been replaced by WSError.
int MLError(MLINK link)
returns a value identifying the last error to occur on link. MLError() returns MLEOK if no error has occurred since the previous call to MLClearError().
Details
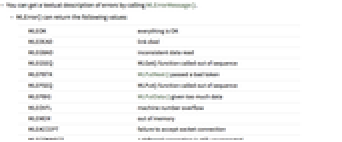
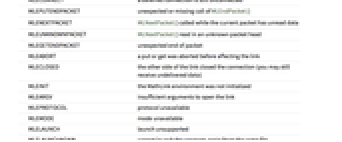
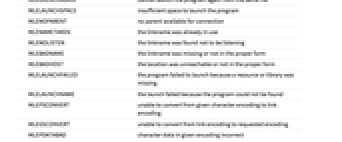
- You can get a textual description of errors by calling MLErrorMessage().
- MLError() can return the following values:
-
MLEOK everything is OK MLEDEAD link died MLEGBAD inconsistent data read MLEGSEQ MLGet() function called out of sequence MLEPBTK MLPutNext() passed a bad token MLEPSEQ MLPut() function called out of sequence MLEPBIG MLPutData() given too much data MLEOVFL machine number overflow MLEMEM out of memory MLEACCEPT failure to accept socket connection MLECONNECT a deferred connection is still unconnected MLEPUTENDPACKET unexpected or missing call of MLEndPacket() MLENEXTPACKET MLNextPacket() called while the current packet has unread data MLEUNKNOWNPACKET MLNextPacket() read in an unknown packet head MLEGETENDPACKET unexpected end of packet MLEABORT a put or get was aborted before affecting the link MLECLOSED the other side of the link closed the connection (you may still receive undelivered data) MLEINIT the MathLink environment was not initialized MLEARGV insufficient arguments to open the link MLEPROTOCOL protocol unavailable MLEMODE mode unavailable MLELAUNCH launch unsupported MLELAUNCHAGAIN cannot launch the program again from the same file MLELAUNCHSPACE insufficient space to launch the program MLENOPARENT no parent available for connection MLENAMETAKEN the linkname was already in use MLENOLISTEN the linkname was found not to be listening MLEBADNAME the linkname was missing or not in the proper form MLEBADHOST the location was unreachable or not in the proper form MLELAUNCHFAILED the program failed to launch because a resource or library was missing MLELAUNCHNAME the launch failed because the program could not be found MLEPSCONVERT unable to convert from given character encoding to link encoding MLEGSCONVERT unable to convert from link encoding to requested encoding MLEPDATABAD character data in given encoding incorrect MLENOTEXE specified file is not a MathLink executable MLESYNCOBJECTMAKE unable to create MathLink synchronization object MLEBACKOUT yield function terminated MathLink operation MLEBADOPTSYM unable to recognize symbol value on link MLEBADOPTSTR unable to recognize string value on link MLENEEDBIGGERBUFFER function call needs bigger buffer argument - MLError() is declared in the MathLink header file mathlink.h.
Examples
Basic Examples (1)
#include "mathlink.h"
/* send the integer 10 to a link */
void f(MLINK lp)
{
if(! MLPutInteger(lp, 10))
{
/* check the possible errors */
switch(MLError(lp))
{
case MLEDEAD:
/* the link died unexpectedly */
break;
case MLECLOSED:
/* the other side closed the link */
break;
case MLEOK:
/* no error occurred */
break;
default:
/* ... */
}
}
}