WSError (C Function)
int WSError(WSLINK link)
returns a value identifying the last error to occur on link. WSError() returns WSEOK if no error has occurred since the previous call to WSClearError().
Details
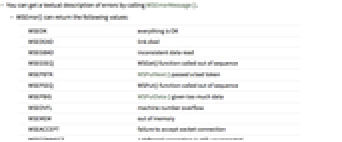
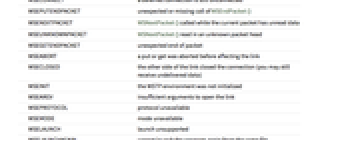
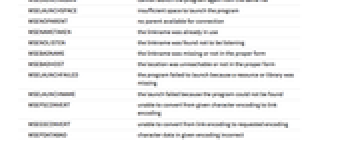
- You can get a textual description of errors by calling WSErrorMessage().
- WSError() can return the following values:
-
WSEOK everything is OK WSEDEAD link died WSEGBAD inconsistent data read WSEGSEQ WSGet() function called out of sequence WSEPBTK WSPutNext() passed a bad token WSEPSEQ WSPut() function called out of sequence WSEPBIG WSPutData() given too much data WSEOVFL machine number overflow WSEMEM out of memory WSEACCEPT failure to accept socket connection WSECONNECT a deferred connection is still unconnected WSEPUTENDPACKET unexpected or missing call of WSEndPacket() WSENEXTPACKET WSNextPacket() called while the current packet has unread data WSEUNKNOWNPACKET WSNextPacket() read in an unknown packet head WSEGETENDPACKET unexpected end of packet WSEABORT a put or get was aborted before affecting the link WSECLOSED the other side of the link closed the connection (you may still receive undelivered data) WSEINIT the WSTP environment was not initialized WSEARGV insufficient arguments to open the link WSEPROTOCOL protocol unavailable WSEMODE mode unavailable WSELAUNCH launch unsupported WSELAUNCHAGAIN cannot launch the program again from the same file WSELAUNCHSPACE insufficient space to launch the program WSENOPARENT no parent available for connection WSENAMETAKEN the linkname was already in use WSENOLISTEN the linkname was found not to be listening WSEBADNAME the linkname was missing or not in the proper form WSEBADHOST the location was unreachable or not in the proper form WSELAUNCHFAILED the program failed to launch because a resource or library was missing WSELAUNCHNAME the launch failed because the program could not be found WSEPSCONVERT unable to convert from given character encoding to link encoding WSEGSCONVERT unable to convert from link encoding to requested encoding WSEPDATABAD character data in given encoding incorrect WSENOTEXE specified file is not a WSTP executable WSESYNCOBJECTMAKE unable to create WSTP synchronization object WSEBACKOUT yield function terminated WSTP operation WSEBADOPTSYM unable to recognize symbol value on link WSEBADOPTSTR unable to recognize string value on link WSENEEDBIGGERBUFFER function call needs bigger buffer argument - WSError() is declared in the WSTP header file wstp.h.
Examples
Basic Examples (1)
#include "wstp.h"
/* send the integer 10 to a link */
void f(WSLINK lp)
{
if(! WSPutInteger(lp, 10))
{
/* check the possible errors */
switch(WSError(lp))
{
case WSEDEAD:
/* the link died unexpectedly */
break;
case WSECLOSED:
/* the other side closed the link */
break;
case WSEOK:
/* no error occurred */
break;
default:
/* ... */
}
}
}