Wolfram Client Library
for Python
Give Python programs access to the power of the Wolfram Language
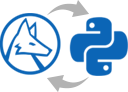
The Wolfram Client Library for Python lets Python programs directly integrate Wolfram Language capabilities. Connect either to a local Wolfram Engine or to the Wolfram Cloud (or a private Wolfram Cloud).
Install the library:
$ pip install wolframclient
Set up your Wolfram Language session:
>>> from wolframclient.evaluation import WolframLanguageSession
>>> from wolframclient.language import wl, wlexpr
>>> session = WolframLanguageSession()
Evaluate any Wolfram Language code from Python:
>>> session.evaluate(wlexpr('Range[5]'))
[1, 2, 3, 4, 5]
Immediately call all 6000+ built-in Wolfram Language functions in Python:
>>> session.evaluate(wl.MinMax([1, -3, 0, 9, 5]))
[-3, 9]
Build up Wolfram Language code directly in Python:
>>> func_squared = wlexpr('#^2 &')
>>> session.evaluate(wl.Map(func_squared, wl.Range(5)))
[1, 4, 9, 16, 25]
Direct support for PIL, Pandas and NumPy libraries:
Create a Pandas DataFrame:
>>> import pandas
>>> df = pandas.DataFrame({'A': [1, 2], 'B': [11, 12]}, index=['id1', 'id2'])
>>> df
A B
id1 1 11
id2 2 12
Apply Wolfram Language function directly to the DataFrame:
>>> session.evaluate(wl.Total(df))
{'A': 3, 'B': 23}
Define native Python functions:
>>> str_reverse = session.function(wl.StringReverse)
>>> str_reverse('abc')
'cba'
Represent Wolfram Language expressions as Python objects:
Use object representation:
>>> wl.Quantity(12, "Hours")
Quantity[12, 'Hours']
Use string representation:
>>> wlexpr('f[x_] := x^2')
(f[x_] := x^2)
Within your Python environment, the Wolfram Client Library for Python lets you:
Access the power of Wolfram algorithms:
Get immediate access to the world's largest integrated algorithmbase:
>>> limit = wlexpr('Limit[x Log[x^2], x -> 0]')
>>> session.evaluate(limit)
0
Access the Wolfram Knowledgebase:
Get the closest ocean:
>>> ocean = wlexpr('GeoNearest[Entity["Ocean"], Here]')
>>> session.evaluate(ocean)
[Entity['Ocean', 'AtlanticOcean']]
Use Wolfram's natural language understanding in Python:
Query Wolfram|Alpha directly in Python:
>>> session.evaluate(wl.WolframAlpha("number of moons of Saturn", "Result"))
62
Terminate the session and release all resources:
>>> session.terminate()