WSBrowseForLinkServices()
int WSBrowseForLinkServices(WSENV e,WSBrowseCallbackFunction f,const char *p,const char *d,void *c,WSServiceRef *r)
ネットワークドメイン d でWSTPサービスのネットワークのブラウズを開始して,非同期にコールバック関数 f を通してサービス状況の適用を通知し,f にコンテキストオブジェクト c とブラウズ操作参照 r を渡す.
詳細
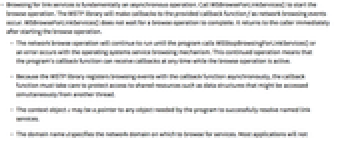
- リンクサービスを探すことは,基本的に非同期操作である. WSBrowseForLinkServices()を呼び出してブラウズ操作を開始する.WSTPライブラリは,ネットワークブラウジングイベントが起ったときに,提供されたコールバック関数 f に対してコールバックを行う.WSBrowseForLinkServices()は,ブラウズ操作が完了するまで待たない.ブラウズ操作が開始してすぐに呼出し側を返す.
- プログラムがWSStopBrowsingForLinkServices()を呼び出すか,オペレーティングシステムのサービスブラウジング構造でエラーが起るかするまで,ネットワークブラウズ操作は続行される.この継続的な操作は,プログラムのコールバック関数がブラウズ操作がアクティブである間にいつでもコールバックを受け取ることが可能であることを意味する.
- WSTPライブラリは,コールバック関数を持つブラウジングイベントを非同期的に登録するので,コールバック関数は,別のスレッドから同時にアクセスされる可能性があるデータ構造等の共有リソースへのアクセスを保護しなければならない.
- コンテキストオブジェクト c は,プログラムが名前付きのリンクサービスをうまく変換するために必要な任意オブジェクトへのポインタである場合がある.
- ドメイン名 d は,サービスを探すネットワークドメインを指定する.アプリケーションの多くは,ドメイン名を指定することはなく,代りにNULLを d に渡し,WSBrowseForLinkServices()がデフォルトのドメイン上でサービスを探してブラウズすべきであることを示す.
- WSStopBrowsingForLinkServices()を使ってブラウズ操作を停止する.
- WSBrowseForLinkServices()は,エラーが起り,ブラウズ操作を再起動しなければならない場合を除き,リンクサービスを1度ブラウズするだけでよい.
- WSBrowseForLinkServices()は,関数が成功した場合には0を,関数が失敗した場合には非零の値を返す.
- エラーの結果としてWSBrowseForLinkServices()から返された非零の値は,wstp.hでのWSEエラーコードとWSError()によって返されるものに対応する.
例題
例 (1)
#include "wstp.h"
void BrowseCallbackFunction(WSENV e, WSServiceRef r, int flag, const char *serviceName, void *context);
void doWSTPServiceBrowsing(WSENV e, const char *protocol)
{
int apiResult;
WSServiceRef r;
apiResult = WSBrowseForLinkServices(env, BrowseCallbackFunction,
protocol, NULL /* For the default browse domain */, NULL, &r);
if(apiResult != 0)
{ /* handle error */}
/* Perform other tasks while browsing occurs */
/* For completeness stop the browse operation, this would normally
be handled at another point in the code */
WSStopBrowsingForLinkServices(e, r);
}
void BrowseCallbackFunction(WSENV e, WSServiceRef r, int flag, const char *serviceName, void *context)
{
if(flag & WSSDADDSERVICE)
{
/* The service named in serviceName has become available
on the network, store the name for use in the program. Don't
forget to include any synchronization mechanisms needed for
ensuring the integrity of shared data structures. */
}
else if(flag & WSSDREMOVESERVICE)
{
/* The service named in serviceName is no longer available
on the network, remove the name from any stored account of
named services on the network. Again, don't forget to make
use of synchronization mechanisms to ensure application integrity. */
}
else if(flag & WSSDBROWSEERROR)
{
/* An error has occurred with the browse operation. When this case happens, the program should use
WSStopBrowsingForLinkServices() to shutdown the browse
operation. An error communicating with the operating system
service discovery mechanisms has occurred and the browse
should be stopped and re-started. In addition, when
WSSDBROWSEERROR occurs, all cached service names should be
released. */
}
}