WSPutRawData (C 関数)
int WSPutRawData(WSLINK link, const unsigned char *d, int l)
長さがl バイトでd からの未加工の文字データあるいは数値データをlink に置く.
詳細
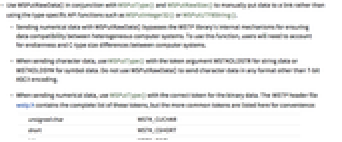
- WSPutRawData()をWSPutType(),WSPutRawSize()と一緒に使うと,WSPutInteger32()あるいはWSPutUTF8String()等の型特定のAPI関数を使わずに手入力でデータをリンクに置くことができる.
- WSPutRawData()を使って数値データを送信すると,異種のコンピュータシステム間のデータの互換性を確保するWSTPライブラリ内部構造を通らずに送信される.この関数を使うためには,ユーザはコンピュータシステム間のエンディアンネスとC言語の型のサイズの違いを考慮する必要がある.
- 文字データを送信する場合は,WSPutType()を文字列データについてはトークン引数WSTKOLDSTRと一緒に,記号データについてはトークン引数WSTKOLDSYMと一緒に使うとよい.WSPutRawData()を使って7ビットのASCIIコード化形式以外の形式の文字データを送信してはならない.
- 数値データを送信する場合は,バイナリデータ用の正しいトークンと一緒にWSPutType()を使うとよい.WSTPヘッダファイルwstp.hにこれらのトークンの完全なリストが含まれているが,ここに便宜上よく使われるトークンを挙げておく.
-
unsigned char WSTK_CUCHAR short WSTK_CSHORT int WSMLTK_CINT long WSTK_CLONG float WSTK_CFLOAT double WSTK_CDOUBLE long double WSTK_CLONGDOUBLE - WSPutRawData()はエラーがあると0を返し,関数が成功するとゼロ以外の値を返す.
- WSError()を使うと,WSPutRawData()が不成功の場合にエラーコードを引き出すことができる.
- WSPutRawData()は,WSTPヘッダファイルwstp.hの中で宣言される.
例題
例 (1)
#include "wstp.h"
/* send a function as raw data to a link */
void f(WSLINK lp)
{
int a = 2, b = 3;
if(! WSPutType(lp, WSTKFUNC))
{ return; /* unable to put the function type to lp */ }
if(! WSPutArgCount(lp, 2))
{ return; /* unable to put the number of arguments to lp */ }
if(! WSPutType(lp, WSTKOLDSYM))
{ return; /* unable to put function head type to lp */ }
if(! WSPutRawSize(lp, 4))
{ return; /* unable to put the size of the symbol to lp */ }
if(! WSPutRawData(lp, (const unsigned char *)"Plus", 4))
{ return; /* unable to put the raw bytes to lp */ }
if(! WSPutType(lp, WSTK_CINT))
{ return; /* unable to put the argument type to lp */ }
if(! WSPutRawSize(lp, sizeof(int)))
{ return; /* unable to put the size of the integer to lp */ }
if(! WSPutRawData(lp, (const unsigned char *)&a, sizeof(a)))
{ return; /* unable to put the raw bytes of a to lp */ }
if(! WSPutType(lp, WSTK_CINT))
{ return; /* unable to put the second argument type to lp */ }
if(! WSPutRawSize(lp, sizeof(int)))
{ return; /* unable to put the size of the integer to lp */ }
if(! WSPutRawData(lp, (const unsigned char *)&b, sizeof(b)))
{ return; /* unable to put the raw bytes of b to lp */ }
}