FindInstance
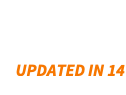
FindInstance[expr,vars]
finds an instance of vars that makes the statement expr be True.
FindInstance[expr,vars,dom]
finds an instance over the domain dom. Common choices of dom are Complexes, Reals, Integers, and Booleans.
FindInstance[expr,vars,dom,n]
finds n instances.
Details and Options
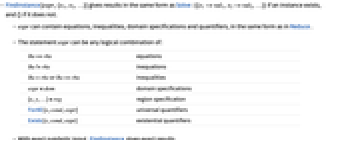
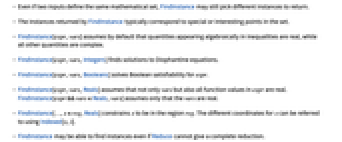
- FindInstance[expr,{x1,x2,…}] gives results in the same form as Solve: {{x1->val1,x2->val2,…}} if an instance exists, and {} if it does not.
- expr can contain equations, inequalities, domain specifications and quantifiers, in the same form as in Reduce.
- The statement expr can be any logical combination of:
-
lhs==rhs equations lhs!=rhs inequations lhs>rhs or lhs>=rhs inequalities expr∈dom domain specifications {x,y,…}∈reg region specification ForAll[x,cond,expr] universal quantifiers Exists[x,cond,expr] existential quantifiers - With exact symbolic input, FindInstance gives exact results.
- Even if two inputs define the same mathematical set, FindInstance may still pick different instances to return.
- The instances returned by FindInstance typically correspond to special or interesting points in the set.
- FindInstance[expr,vars] assumes by default that quantities appearing algebraically in inequalities are real, while all other quantities are complex.
- FindInstance[expr,vars,Integers] finds solutions to Diophantine equations.
- FindInstance[expr,vars,Booleans] solves Boolean satisfiability for expr.
- FindInstance[expr,vars,Reals] assumes that not only vars but also all function values in expr are real. FindInstance[expr&&vars∈Reals,vars] assumes only that the vars are real.
- FindInstance[…,x∈reg,Reals] constrains x to be in the region reg. The different coordinates for x can be referred to using Indexed[x,i].
- FindInstance may be able to find instances even if Reduce cannot give a complete reduction.
- By default, every time you run FindInstance with a given input, it will return the same output.
- FindInstance[expr,vars,dom,n] will return a shorter list if the total number of instances is less than n.
- The following options can be given:
-
Method Automatic method to use Modulus 0 modulus to assume for integers RandomSeeding 1234 how to seed randomness WorkingPrecision Infinity precision to use in internal computations
Examples
open allclose allBasic Examples (6)
Scope (57)
Complex Domain (11)
A univariate polynomial equation:
Five roots of a polynomial of a high degree:
A multivariate polynomial equation:
Systems of polynomial equations and inequations:
This gives three solution instances:
If there are no solutions FindInstance returns an empty list:
If there are fewer solutions than the requested number, FindInstance returns all solutions:
Five out of a trillion roots of a polynomial system:
In this case there is no solution:
A solution in terms of transcendental Root objects:
Five roots of an unrestricted equation:
Real Domain (13)
A univariate polynomial equation:
A univariate polynomial inequality:
A multivariate polynomial equation:
A multivariate polynomial inequality:
Systems of polynomial equations and inequalities:
If there are no solutions FindInstance returns an empty list:
If there are fewer solutions than the requested number, FindInstance returns all solutions:
A quantified polynomial system:
A solution in terms of transcendental Root objects:
Integer Domain (12)
A linear system of equations and inequalities:
A univariate polynomial equation:
A univariate polynomial inequality:
If there are fewer solutions than the requested number, FindInstance returns all solutions:
A bounded system of equations and inequalities:
A high-degree system with no solution:
Modular Domains (5)
Finite Field Domains (4)
Mixed Domains (3)
Mixed real and complex variables:
Find a real value of and a complex value of
for which
is real and less than
:
An inequality involving Abs[z]:
Geometric Regions (9)
Find instances in basic geometric regions in 2D:
Find instances in basic geometric regions in 3D:
Find a point in the projection of a region:
A parametrically defined region:
Regions dependent on parameters:
Find values of parameters ,
, and
for which the circles contain the given points:
Options (3)
RandomSeeding (1)
Finding instances often involves random choice from large solution sets:
By default, FindInstance chooses the same solutions each time:
Use RandomSeedingAutomatic to generate potentially new instances each time:
WorkingPrecision (1)
Finding an exact solution to this problem is hard:
With a finite WorkingPrecision, FindInstance is able to find an approximate solution:
Applications (11)
Geometric Problems (6)
The region ℛ is a subset of if is empty. Show that Disk[{0,0},{2,1}] is a subset of Rectangle[{-2,-1},{2,1}]:
Show that Rectangle[] is a not a subset of Disk[{0,0},7/5]:
Show that Cylinder[]⊆Ball[{0,0,0},2]:
Show that Cylinder[]⊈Ball[{0,0,0},7/5]:
Find a point in the intersection of two regions:
Boolean Problems (2)
Prove that a statement is a tautology:
This can be proven with TautologyQ as well:
Show that a statement is not a tautology; get a counterexample:
This can be done with SatisfiabilityInstances as well:
Properties & Relations (10)
Solution instances satisfy the input system:
Use RootReduce to prove that algebraic numbers satisfy equations:
When there are no solutions, FindInstance returns an empty list:
If there are fewer solutions than the requested number, FindInstance returns all solutions:
To get a complete description of the solution set use Reduce:
To get a generic solution of a system of complex equations use Solve:
Solving a sum of squares representation problem:
Use SquaresR to find the number of solutions to sum of squares problems:
Solving a sum of powers representation problem:
Use PowersRepresentations to enumerate all solutions:
Find instances satisfying a Boolean statement:
Use SatisfiabilityInstances to obtain solutions represented as Boolean vectors:
FindInstance shows that the polynomial is non-negative:
Use PolynomialSumOfSquaresList to represent as a sum of squares:
The Motzkin polynomial is non-negative, but is not a sum of squares:

Text
Wolfram Research (2003), FindInstance, Wolfram Language function, https://reference.wolfram.com/language/ref/FindInstance.html (updated 2024).
CMS
Wolfram Language. 2003. "FindInstance." Wolfram Language & System Documentation Center. Wolfram Research. Last Modified 2024. https://reference.wolfram.com/language/ref/FindInstance.html.
APA
Wolfram Language. (2003). FindInstance. Wolfram Language & System Documentation Center. Retrieved from https://reference.wolfram.com/language/ref/FindInstance.html