Real-time data acquisition
Acquire real-time data from a sensor
Introduction
Microcontroller boards can typically communicate with both small sensors and personal computers, and sometimes even over WiFi. Thus they are very handy in acquiring real-world data from sensors when no direct interface exists between the sensor and the computer.
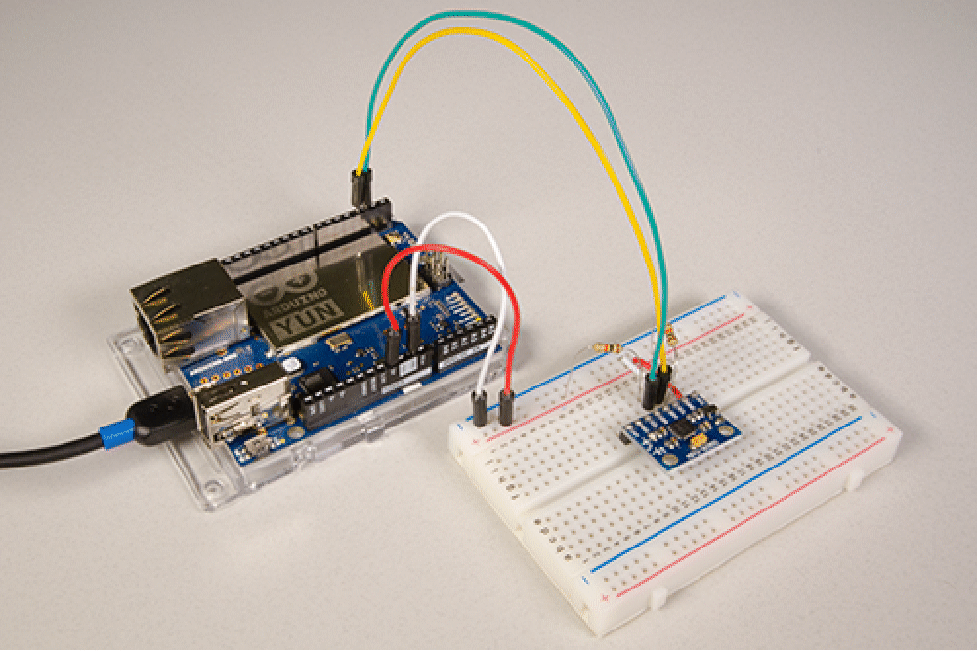
In this project, we will stream data from a MPU-6050 sensor to a Wolfram Data Drop repository using an Arduino Yun microcontroller board. The MPU-6050 is an I2C device with a 3-axis accelerometer, a 3-axis gyroscope, and a temperature sensor. The Arduino Yun uploads the data over the WiFi interface. The microcontroller will be programmed to initialize the sensor, acquire data from it, and upload the data to the repository. The real-time data can then be accessed from the repository.
Electrical wirings
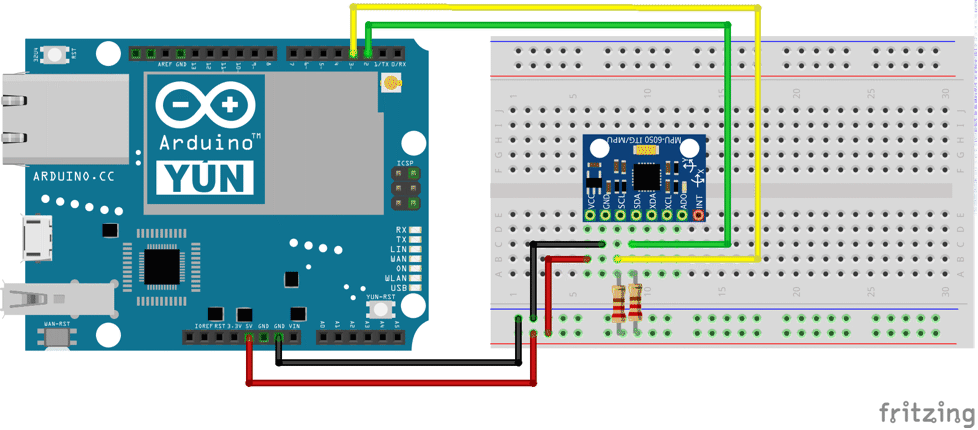
I2C
We will first setup the microcontroller to receive data from the MPU-6050 sensor.
The I2C address of the MPU-6050 .
From the datasheet we understand that the 3 accelerometer, 1 thermostat, and 3 gyrospope readings are stored in two bytes each starting from address 0x3B. Thus the microcontroller has to read a total of 14 bytes, and will send an acknowledgement after each reading except the last one.
The sensor needs to be also initialized before it can send out the data.
The complete I2C specifications for the sensor.
The accelerations and angular velocities are sent as 2's complements. Create a utility to converts to decimal from the 2’s complement value.
A second master utility to handle all measurements based on if they need such a conversion.
The master utility function is used to convert the 14 bytes to 7 decimal values.
The 7 values are obtained from the same data line SDA, which is pin 2 on the Arduino Yun.
A model to convert the acceleration to units of m/s2, temperature to °C, and angular velocity to rad/s from the decimal values.
Databin
We will now create the databin and setup the output channels to upload the data using Arduino’s bridge library to the data drop repository.
Create the databin.
The location of the bridge library that is needed to upload values to the databin.
The header file along with the utilities and initialization code.
The RESTful API code that adds the data to the databin.
The code snippet that uploads the first data entry.
Embed the code
Load the package.
Since the core Arduino libraries are being used by the Bridge library we will also use it for the timer and CDC functionality.
The specifications of the MPU-6050 I2C device and the Bridge library are needed for the microcontroller to receive and send data. Use the ‘-w’ option to supress the harmless compiler warning.
Visualize the data
Create a function that to plot the acceleration and angular velocity data.
Visualize the acceleration, angular velocity, and temperature data.
In conclusion, we have seen how to get I2C data from a sensor, upload it to a databin in the Wolfram Data Drop repository, and visualize the values in the databin.
More things to try
- Explore other options to vizualize and anlalyze the data.
- Implement a filter than smooth the data.
- Send the data using the channel framework.
- Send the data directly to the computer over a virtual COM port.