Part 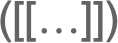
expr[[i]] or Part[expr,i]
gives the i part of expr.
expr[[-i]]
counts from the end.
expr[[i,j,…]] or Part[expr,i,j,…]
is equivalent to expr[[i]][[j]]….
expr[[{i1,i2,…}]]
gives a list of the parts i1, i2, … of expr.
expr[[m;;n]]
gives parts m through n.
expr[[m;;n;;s]]
gives parts m through n in steps of s.
expr[["key"]]
gives the value associated with the key "key" in an association expr.
expr[[Key[k]]]
gives the value associated with an arbitrary key k in the association expr.
Details
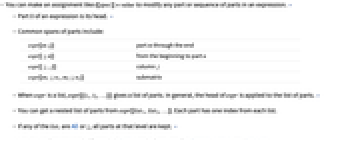
- You can make an assignment like t[[spec]]=value to modify any part or sequence of parts in an expression. »
- Part 0 of an expression is its head. »
- Common spans of parts include:
-
expr[[m;;]] part m through the end expr[[;;n]] from the beginning to part n expr[[;;,j]] column j expr[[m1;;n1,m2;;n2]] submatrix - When expr is a list, expr[[{i1,i2,…}]] gives a list of parts. In general, the head of expr is applied to the list of parts. »
- You can get a nested list of parts from expr[[list1,list2,…]]. Each part has one index from each list.
- If any of the listi are All or ;;, all parts at that level are kept. »
- Notice that lists are used differently in Part than in functions like Extract, MapAt, and Position.
- If expr is a SparseArray object or a structured array such as QuantityArray or SymmetrizedArray, expr[[…]] gives the parts in the corresponding ordinary array. »
- The form expr[["key"]] can be used to extract a value from an association whose key is a string. expr[[Key[k]]] can be used to extract values with any keys.
- "key" and Key[k] can appear anywhere in the specification of parts.
- In StandardForm and InputForm, expr[[spec]] can be input as expr〚spec〛.
- 〚 and 〛 can be entered as
[[
and
]]
or \[LeftDoubleBracket] and \[RightDoubleBracket].
- In StandardForm, expr[[spec]] can be input as expr[[spec]] or expr〚spec〛.
Background & Context
- Part is a structural function that gives a specified indexed part of an expression. The expression Part[expr,i] is commonly represented using the shorthand syntax expr[[i]] or expr〚i〛. Part can be used to pick out parts of lists, a sequence of parts, elements of matrices, rows and columns of matrices, and so forth. Part can also be used to assign values to parts using Set, e.g. list[[k]]=newValue.
- Although Part is most commonly used with lists, it works with expressions of any kind. When using Part, parts of expressions may be specified using indices, lists of indices, Span expressions, or All.
- Useful functions that perform common special cases of Part include First, Last, Take, Drop, Rest, and Most. Position can be used to find the positions in an expression at which specified content appears. Extract is a more specialized function that extracts the part of an expression at the position specified by a given list, while the analogous function Delete removes an element from a specified position.
- A small number of functions contain parts that cannot be accessed or divided into subexpressions using Part or related functions. The most common cases are Complex and Rational, where for example Complex[1,2] is the internal representation for numbers such as 1+2 and Rational[1,2] is the internal representation for numbers such as 1/2. Functions having this property are said to be atomic, and they return True when AtomQ is applied to them.
Examples
open allclose allBasic Examples (6)
Scope (23)
Single-Level Specifications (7)
Select several parts, maintaining the head:
Obtain a list of parts, including repeats:
Parts 1 through third-to-last:
Parts 3 through third-to-last extracted in steps of 2:
Parts extracted in steps of 2 starting at the beginning:
The first part of an Association object:
The part associated with the key 1:
Multilevel Specifications (7)
This can be understood as first extracting the middle row:
This is followed by extracting the third column from that row:
The second column of a matrix:
Pick out parts 2 and 3 of parts 1 and 3:
Extract a subexpression from an association:
Extract several subexpressions:
Use a mixture of Key-based and numeric indices:
Extract deep parts of arbitrary expressions:
For SparseArray objects, Part gives the parts in the corresponding ordinary array:
Rows or columns are represented as sparse vectors:
For structured arrays, Part gives the parts in the corresponding ordinary array:
Parts from a QuantityArray are a Quantity object with units:
Assignments (5)
Input Forms (4)
Enter in FullForm:
Enter a Span specification using FullForm:
Use the special characters \[LeftDoubleBracket]and \[RightDoubleBracket]:
Applications (6)
Pick out the first solution from an equation:
Pick out all solutions for a univariate equation:
Go through the first 1000 primes, and count how many lie in each possible "mod 10 bin":
Another way to get the same result:
Verify the result with a direct computation using InversePermutation:
Pick out parts cyclically by using Mod with offset 1:
Properties & Relations (10)
The zeroth part of an expression is its head:
Heads in the original expression are preserved when using a list of parts specification:
This is true even when using a multilevel specification:
Part[expr,{}] yields Head[expr][]:
For string keys, Key["key"] and "key" are equivalent:
This is not true of general keys:

Part[expr] gives expr:
Assigning to part 0 is effectively a form of Apply:
Perform the same operation using @@ (Apply):
Part[expr,m;;n] is effectively equivalent to Take[expr,{m,n}]:
Similarly, Part[expr,m;;n;;s] is effectively equivalent to Take[expr,{m,n,s}]:
Part[expr,i,j,…] is effectively equivalent to Extract[expr,{i,j,…}]:
Part can extract rectangular blocks:
Extract can return lists of parts at arbitrary positions:
Permute and Part interpret permutations differently:
The two interpretations are related by InversePermutation:
Possible Issues (5)
Position does not return part specifications in a form that can immediately be used by Part:
Extract extracts parts specified in the way returned by Position:
When using a list of parts, successive part extraction is not always equivalent to direct part extraction:

If an expression contains held parts, extraction may cause them to evaluate:
This can lead to a difference between direct part extraction and successive part extraction:
Only parts that already exist can be reassigned:

Create a shared variable whose value is a large matrix:
Accessing its elements causes the whole matrix to be transferred to the subkernels repeatedly:
Use Unevaluated to allow the special code for parts of shared variables to see the variable:
Text
Wolfram Research (1988), Part, Wolfram Language function, https://reference.wolfram.com/language/ref/Part.html (updated 2014).
CMS
Wolfram Language. 1988. "Part." Wolfram Language & System Documentation Center. Wolfram Research. Last Modified 2014. https://reference.wolfram.com/language/ref/Part.html.
APA
Wolfram Language. (1988). Part. Wolfram Language & System Documentation Center. Retrieved from https://reference.wolfram.com/language/ref/Part.html