WSTP Development in C (Windows)
Overview | Building WSTP Programs |
Supported Development Platforms | Running WSTP Programs |
Installing the WSTP Components | Troubleshooting |
Recommended Installation
SystemAdditions Installation for All Compilers
CompilerAdditions Installation for Microsoft Compilers
Visual Studio 2012
1. Navigate to the Windows\CompilerAdditions folder (on 64-bit Windows systems you will use the Windows-x86-64\CompilerAdditions\ folder) in the Mathematica installation. You can open a window containing these folders by running the following command.
explorer "C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows\CompilerAdditions"
The word explorer is optional but the quotation marks are not.
2. Copy the files wstp32i4.lib, wstp32i4m.lib, and wstp32i4s.lib to C:\Program Files\Microsoft Visual Studio 11\VC\lib. Note that for 64-bit Windows systems, these files will be named wstp64i4*. Copy wstp.h to C:\Program Files\Microsoft Visual Studio 11\VC\include. Finally, copy wsprep.exe to C:\Program Files\Microsoft Visual Studio 11\VC\bin. Note that on 64-bit Windows systems, you will change the destination paths to C:\Program Files (x86)\Microsoft Visual Studio 11\VC\lib\AMD64, C:\Program Files (x86)\Microsoft Visual Studio 11\VC\include, and C:\Program Files (x86)\Microsoft Visual Studio 11\VC\bin.
Visual Studio 2010
1. Navigate to the Windows\CompilerAdditions folder (on 64-bit Windows systems you will use the Windows-x86-64\CompilerAdditions\ folder) in the Mathematica installation. You can open a window containing these folders by running the following command.
explorer "C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows\CompilerAdditions"
The word explorer is optional but the quotation marks are not.
2. Copy the files wstp32i4.lib, wstp32i4m.lib, and wstp32i4s.lib to C:\Program Files\Microsoft Visual Studio 11\VC\lib. Note that for 64-bit Windows systems, these files will be named wstp64i4*. Copy wstp.h to C:\Program Files\Microsoft Visual Studio 11\VC\include. Finally, copy wsprep.exe to C:\Program Files\Microsoft Visual Studio 11\VC\bin. Note that on 64-bit Windows systems, you will change the destination paths to C:\Program Files (x86)\Microsoft Visual Studio 11\VC\lib\AMD64, C:\Program Files (x86)\Microsoft Visual Studio 11\VC\include, and C:\Program Files (x86)\Microsoft Visual Studio 11\VC\bin.
Elements of the WSTP Developer Kit
WSTP Shared Libraries and Header Files
SystemAdditions Directory
wstp32i4.dll/wstp64i4.dll
The WSTP Devices
char* argv[] = {"-linkname", "foo", "-linkprotocol", "SharedMemory", "-linkmode", "connect"};
link = WSOpenArgv( stdenv, argv, argv + 6, 0);
char* argv[] = {"-linkname", "6000", "-linkprotocol", "TCPIP", "-linkmode", "connect"};
link = WSOpenArgv( stdenv, argv, argv + 6, 0);
char* argv[] = {"-linkname", "abcdef_ipq", "-linkprotocol", "IntraProcess", "-linkmode", "connect"};
link = WSOpenArgv(stdenv, argv, argv + 6, 0);
char *argv[] = {"-linkname", "abcdef_ipq", "-linkprotocol", "IntraProcess", "-linkmode", "listen"};
link = WSOpenArgv(stdenv, argv, argv + 6, 0);
char* argv[] = {"-linkname", "foo", "-linkprotocol", "FileMap", "-linkmode", "connect"};
link = WSOpenArgv( stdenv, argv, argv + 6, 0);
char* argv[] = {"-linkname", "6000", "-linkprotocol", "TCP", "-linkmode", "connect"};
link = WSOpenArgv( stdenv, argv, argv + 6, 0);
CompilerAdditions Directory
Visual Studio 2012
CompilerAdditions\wsprep.exe
Visual Studio 2010
CompilerAdditions\wstp.h
CompilerAdditions\wstp*i*.lib
CompilerAdditions\wsprep.exe
PrebuiltExamples Directory
WSTPExamples Directory
Alternative Components Directory
WSTP Versioning
Strategy
Using WSTP Template Files
Building WSTP Programs with Microsoft Visual Studio
Using the Command Line Tools
Visual Studio 2012
1. Start a command prompt window by running Start ▶ All Programs ▶ Microsoft Visual Studio 2012 ▶ Visual Studio Tools ▶ VS2012 x86 Native Tools Command Prompt (On 64-bit Windows, use Start ▶ All Programs ▶ Microsoft Visual Studio 2012 ▶ Visual Studio Tools ▶ VS2012 x64 Cross Tools Command Prompt).
2. Change to the WSTPExamples directory.
C:
cd "C:\Program Files\Wolfram Research\Mathematica\11.0\"
cd SystemFiles\Links\WSTP\DeveloperKit\Windows\WSTPExamples\
On 64-bit Windows, use the following commands.
C:
cd "C:\Program Files\Wolfram Research\Mathematica\11.0\"
cd SystemFiles\Links\WSTP\DeveloperKit\Windows-x86-64\WSTPExamples\
On some versions of Windows, you may need to copy the contents of the WSTPExamples directory to a folder in the file system for which you have permissions for writing.
3. Type the following five commands.
SET CL=/nologo /c /DWIN32 /D_WINDOWS /W3 /O2 /DNDEBUG
SET LINK=/NOLOGO /SUBSYSTEM:windows /INCREMENTAL:no /PDB:NONE kernel32.lib user32.lib gdi32.lib
WSPREP addtwo.tm -o addtwotm.c
CL addtwo.c addtwotm.c
LINK addtwo.obj addtwotm.obj wstp32i4m.lib /OUT:addtwo.exe
On 64-bit Windows, use the following command in place of the last command.
LINK addtwo.obj addtwotm.obj wstp64i4m.lib /OUT:addtwo.exe
4. Start a command prompt window by running Start ▶ All Programs ▶ Microsoft Visual Studio 2012 ▶ Visual Studio Tools ▶ VS2012 x86 Native Tools Command Prompt (On 64-bit Windows, use Start ▶ All Programs ▶ Microsoft Visual Studio 2012 ▶ Visual Studio Tools ▶ VS2012 x64 Cross Tools Command Prompt).
5. Change to the WSTPExamples directory.
C:
cd "C:\Program Files\Wolfram Research\Mathematica\11.0\"
cd AddOns\WSTP\DeveloperKit\Windows\WSTPExamples\
On 64-bit Windows, use the following commands.
C:
cd "C:\Program Files\Wolfram Research\Mathematica\11.0\"
cd SystemFiles\Links\WSTP\DeveloperKit\Windows-x86-64\WSTPExamples\
On some versions of Windows, you may need to copy the contents of the WSTPExamples directory to a folder in the file system for which you have permissions for writing.
6. Type the following four commands.
SET CL=/nologo /c /DWIN32 /D_CONSOLE /W3 /O2 /DNDEBUG
SET LINK=/NOLOGO /SUBSYSTEM:console /INCREMENTAL:no /PDB:NONE kernel32.lib user32.lib
CL factor.c
LINK factor.obj wstp32i4m.lib /OUT:factor.exe
On 64-bit Windows, use the following command in place of the last command.
LINK factor.obj wstp64i4m.lib /OUT:factor.exe
7. Follow steps 1–3 in "Building a WSTP Program to Be Called by the Wolfram Language Kernel", replacing the commands in step 3 with the following.
SET CL=/nologo /c /DWIN32 /D_WINDOWS /W3 /Z7 /Od /D_DEBUG
SET LINK=/NOLOGO /SUBSYSTEM:windows /DEBUG /PDB:NONE /INCREMENTAL:no kernel32.lib user32.lib gdi32.lib
WSPREP addtwo.tm -o addtwotm.c
CL addtwo.c addtwotm.c
LINK addtwo.obj addtwotm.obj wstp32i4m.lib /OUT:addtwo.exe
On 64-bit Windows, use the following command in place of the last command.
LINK addtwo.obj addtwotm.obj wstp64i4m.lib /OUT:addtwo.exe
8. Start Microsoft Visual Studio.
9. From the File menu, choose Open ▶ Project/Solution.
The Open Project dialog box appears.
10. Select Exe Project Files (*.exe) from the file type drop-down list to display .exe files in the file chooser pane.
11. Select the drive and directory containing addtwo.exe.
12. Select addtwo.exe and click the Open button.
13. To start debugging, press F5 or choose the Start Debugging command under the Debug menu.
When you are done debugging and close the project solution, you will be asked if you want to save the new solution associated with addtwo.exe. Choose OK if you want to retain your breakpoints and other debugger settings.
14. Follow steps 1–3 in "Building a WSTP Program That Calls the Wolfram Language Kernel", replacing the commands in step 3 with the following.
SET CL=/nologo /c /DWIN32 /D_CONSOLE /W3 /Z7 /Od /D_DEBUG
SET LINK=/NOLOGO /SUBSYSTEM:console /INCREMENTAL:no /PDB:NONE /DEBUG kernel32.lib user32.lib
CL factor.c
LINK factor.obj wstp32i4m.lib /OUT:factor.exe
On 64-bit Windows, use the following command in place of the last command.
LINK factor.obj wstp64i4m.lib /OUT:factor.exe
15. Start Microsoft Visual Studio.
16. From the File menu, choose Open ▶ Project/Solution.
The Open Project dialog box appears.
17. Select Exe Project Files (*.exe) from the file type drop-down list to display .exe files in the file chooser pane.
18. Select the drive and directory containing factor.exe.
19. Select factor.exe and click the Open button.
20. From the Project menu, choose Properties.
The project settings page appears.
21. Under the Parameters section, click in the Arguments text box and type: -linklaunch.
23. To start debugging, press F5 or choose the Start Debugging command under the Debug menu.
24. When WSOpenArgcArgv() is executed, the Choose a WSTP Program to Launch dialog box appears. Open MathKernel.exe.
When you are done debugging and close the project solution, you will be asked if you want to save the new solution associated with factor.exe. Choose OK if you want to retain your breakpoints and other debugger settings.
Visual Studio 2010
1. Start a command prompt window by running Start ▶ All Programs ▶ Microsoft Visual Studio 2010 ▶ Visual Studio Tools ▶ Visual Studio Command Prompt (2010) (On 64-bit Windows, use Start ▶ All Programs ▶ Microsoft Visual Studio 2010 ▶ Visual Studio Tools ▶ Visual Studio x64 Win64 Command Prompt (2010)).
2. Change to the WSTPExamples directory.
C:
cd "C:\Program Files\Wolfram Research\Mathematica\11.0\"
cd SystemFiles\Links\WSTP\DeveloperKit\Windows\WSTPExamples\
On 64-bit Windows, use the following commands.
C:
cd "C:\Program Files\Wolfram Research\Mathematica\11.0\"
cd SystemFiles\Links\WSTP\DeveloperKit\Windows-x86-64\WSTPExamples\
On some versions of Windows, you may need to copy the contents of the WSTPExamples directory to a folder in the file system for which you have permissions for writing.
3. Type the following five commands.
SET CL=/nologo /c /DWIN32 /D_WINDOWS /W3 /O2 /DNDEBUG
SET LINK=/NOLOGO /SUBSYSTEM:windows /INCREMENTAL:no /PDB:NONE kernel32.lib user32.lib gdi32.lib
WSPREP addtwo.tm -o addtwotm.c
CL addtwo.c addtwotm.c
LINK addtwo.obj addtwotm.obj wstp32i4m.lib /OUT:addtwo.exe
On 64-bit Windows, use the following command in place of the last command.
LINK addtwo.obj addtwotm.obj wstp64i4m.lib /OUT:addtwo.exe
4. Start a command prompt window by running Start ▶ All Programs ▶ Microsoft Visual Studio 2010 ▶ Visual Studio Tools ▶ Visual Studio Command Prompt (2010) (On 64-bit Windows, use Start ▶ All Programs ▶ Microsoft Visual Studio 2010 ▶ Visual Studio Tools ▶ Visual Studio x64 Win64 Command Prompt (2010)).
5. Change to the WSTPExamples directory.
C:
cd "C:\Program Files\Wolfram Research\Mathematica\11.0\"
cd AddOns\WSTP\DeveloperKit\Windows\WSTPExamples\
On 64-bit Windows, use the following commands.
C:
cd "C:\Program Files\Wolfram Research\Mathematica\11.0\"
cd SystemFiles\Links\WSTP\DeveloperKit\Windows-x86-64\WSTPExamples\
On some versions of Windows, you may need to copy the contents of the WSTPExamples directory to a folder in the file system for which you have permissions for writing.
6. Type the following four commands.
SET CL=/nologo /c /DWIN32 /D_CONSOLE /W3 /O2 /DNDEBUG
SET LINK=/NOLOGO /SUBSYSTEM:console /INCREMENTAL:no /PDB:NONE kernel32.lib user32.lib
CL factor.c
LINK factor.obj wstp32i4m.lib /OUT:factor.exe
On 64-bit Windows, use the following command in place of the last command.
LINK factor.obj wstp64i4m.lib /OUT:factor.exe
7. Follow steps 1–3 in "Building a WSTP Program to Be Called by the Wolfram Language Kernel", replacing the commands in step 3 with the following.
SET CL=/nologo /c /DWIN32 /D_WINDOWS /W3 /Z7 /Od /D_DEBUG
SET LINK=/NOLOGO /SUBSYSTEM:windows /DEBUG /PDB:NONE /INCREMENTAL:no kernel32.lib user32.lib gdi32.lib
WSPREP addtwo.tm -o addtwotm.c
CL addtwo.c addtwotm.c
LINK addtwo.obj addtwotm.obj wstp32i4m.lib /OUT:addtwo.exe
On 64-bit Windows, use the following command in place of the last command.
LINK addtwo.obj addtwotm.obj wstp64i4m.lib /OUT:addtwo.exe
8. Start Microsoft Visual Studio.
9. From the File menu, choose Open ▶ Project/Solution.
The Open Project dialog box appears.
10. Select Exe Project Files (*.exe) from the file type drop-down list to display .exe files in the file chooser pane.
11. Select the drive and directory containing addtwo.exe.
12. Select addtwo.exe and click the Open button.
13. To start debugging, press F5 or choose the Start Debugging command under the Debug menu.
When you are done debugging and close the project solution, you will be asked if you want to save the new solution associated with addtwo.exe. Choose OK if you want to retain your breakpoints and other debugger settings.
14. Follow steps 1–3 in "Building a WSTP Program That Calls the Wolfram Language Kernel", replacing the commands in step 3 with the following.
SET CL=/nologo /c /DWIN32 /D_CONSOLE /W3 /Z7 /Od /D_DEBUG
SET LINK=/NOLOGO /SUBSYSTEM:console /INCREMENTAL:no /PDB:NONE /DEBUG kernel32.lib user32.lib
CL factor.c
LINK factor.obj wstp32i4m.lib /OUT:factor.exe
On 64-bit Windows, use the following command in place of the last command.
LINK factor.obj wstp64i4m.lib /OUT:factor.exe
15. Start Microsoft Visual Studio.
16. From the File menu, choose Open ▶ Project/Solution.
The Open Project dialog box appears.
17. Select Exe Project Files (*.exe) from the file type drop-down list to display .exe files in the file chooser pane.
18. Select the drive and directory containing factor.exe.
19. Select factor.exe and click the Open button.
20. From the Project menu, choose Properties.
The project settings page appears.
21. Under the Parameters section, click in the Arguments text box and type: -linklaunch.
23. To start debugging, press F5 or choose the Start Debugging command under the Debug menu.
24. When WSOpenArgcArgv() is executed, the Choose a WSTP Program to Launch dialog box appears. Open MathKernel.exe.
When you are done debugging and close the project solution, you will be asked if you want to save the new solution associated with factor.exe. Choose OK if you want to retain your breakpoints and other debugger settings.
Short Summary of Compiler Switches
switch | action |
/nologo | do not display the copyright notice |
/W3 | display extended warnings |
/Z7 | store debugging information in the object files |
/Zi | store debugging information in a separate project database file |
/Fdaddtwo.pdb | specify name of the project database file—used with /Zi |
/Od |
turn off optimization (default)
|
/O2 | optimizer prefers faster code over smaller code |
/D | defines used by some standard header files and wstp.h |
/c | compile only without linking |
@filename | read more command line arguments from the file |
CFLAGS | environment variable containing more command line arguments |
Short Summary of Linker Switches
switch | action |
/NOLOGO | do not display the copyright notice |
/DEBUG | store debugging information in the executable or project database |
/PDB:NONE | store debugging information in the executable—used with /DEBUG |
/PDB:addtwo.pdb | override the default name for the project database |
/OUT:addtwo.exe | name the output file |
/INCREMENTAL:no | links more slowly but keeps things smaller and neater |
/SUBSYSTEM:windows |
the application does not need a console because it creates its own windows (default when
WinMain()
is defined)
|
/SUBSYSTEM:console |
a console is provided (default when
main()
is defined)
|
Standard System Libraries
import library | base system services |
kernel32.lib |
base OS support such as the file system, interprocess communication, process control, memory, and the console
|
advapi32.lib | support for security and Registry calls |
import library | GUI system services |
user32.lib |
support for user interface elements such as windows, messages, menus, controls, and dialog boxes
|
gdi32.lib | support for drawing text and graphics |
winspool.lib | support for printing and print jobs |
comdlg32.lib | support for the common dialogs such as those for opening and saving files and printing |
import library | shell system services |
shell32.lib |
support for drag and drop, associations between executables and filename extensions, and icon extraction from executables
|
import library | OLE system services |
ole32.lib | support OLE v2 .1 |
oleaut32.lib | support for OLE automation |
uuid.lib |
support for universally unique identifiers used in OLE and RPC (static library)
|
import library | database system services |
odbc32.lib | access to database management systems through ODBC |
odbccp32.lib | ODBC setup and administration |
Using the Program Build Utility NMAKE
Visual Studio 2012
1. Using a text editor, create a file containing the following text.
# addtwo.mak a makefile for building the addtwo.exe example program
CFLAGS = /nologo /c /W3 /Z7 /Od /DWIN32 /D_DEBUG /D_WINDOWS
# Linking against gdi32.lib for access to windowing mechanisms
LFLAGS = /DEBUG /PDB:NONE /NOLOGO /SUBSYSTEM:windows /INCREMENTAL:no kernel32.lib user32.lib gdi32.lib
# Uncomment the value below for working on a 64-bit Windows system
# PLATFORM = WIN64
PLATFORM = WIN32
!if "$(PLATFORM)" == "WIN32"
LIBFILE = wstp32i4m.lib
!else
LIBFILE = wstp64i4m.lib
!endif
addtwo.exe : addtwo.obj addtwotm.obj
LINK addtwo.obj addtwotm.obj $(LIBFILE) /OUT:addtwo.exe @<<
$(LFLAGS)
<<
addtwo.obj : addtwo.c
CL @<< addtwo.c
$(CFLAGS)
<<
addtwotm.obj : addtwotm.c
CL @<< addtwotm.c
$(CFLAGS)
<<
# Need to call wsprep to preprocess WSTP template
addtwotm.c : addtwo.tm
wsprep addtwo.tm -o addtwotm.c
2. Save the file as addtwo.mak in the WSTPExamples directory.
3. Start a command prompt window by running Start ▶ All Programs ▶ Microsoft Visual Studio 2012 ▶ Visual Studio Tools ▶ VS2012 x86 Native Tools Command Prompt (On 64-bit Windows, use Start ▶ All Programs ▶ Microsoft Visual Studio 2012 ▶ Visual Studio Tools ▶ VS2012 x64 Cross Tools Command Prompt).
4. Change to the addtwo directory.
5. Type the following command.
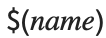
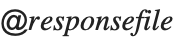
6. Using a text editor, create a file containing the following text.
# factor.mak a makefile for building the factor.exe example program
# This makefile builds a console program
CFLAGS = /nologo /c /MLd /W3 /Z7 /Od /DWIN32 /D_DEBUG /D_CONSOLE
LFLAGS = /DEBUG /PDB:NONE /NOLOGO /SUBSYSTEM:console /INCREMENTAL:no kernel32.lib user32.lib
# Uncomment the value below for working on a 64-bit Windows system
# PLATFORM = WIN64
PLATFORM = WIN32
!if "$(PLATFORM)" == "WIN32"
LIBFILE = wstp32i4m.lib
!else
LIBFILE = wstp64i4m.lib
!endif
factor.exe : factor.obj
LINK factor.obj $(LIBFILE) /OUT:factor.exe @<<
$(LFLAGS)
<<
factor.obj : factor.c
CL @<< factor.c
$(CFLAGS)
<<
7. Save the file as factor.mak in the WSTPExamples directory.
8. Start a command prompt window by running Start ▶ All Programs ▶ Microsoft Visual Studio 2012 ▶ Visual Studio Tools ▶ VS2012 x86 Native Tools Command Prompt (On 64-bit Windows, use Start ▶ All Programs ▶ Microsoft Visual Studio 2012 ▶ Visual Studio Tools ▶ VS2012 x64 Cross Tools Command Prompt).
9. Change to the factor directory.
10. Type the following two commands.
Visual Studio 2010
1. Using a text editor, create a file containing the following text.
# addtwo.mak a makefile for building the addtwo.exe example program
CFLAGS = /nologo /c /W3 /Z7 /Od /DWIN32 /D_DEBUG /D_WINDOWS
# Linking against gdi32.lib for access to windowing mechanisms
LFLAGS = /DEBUG /PDB:NONE /NOLOGO /SUBSYSTEM:windows /INCREMENTAL:no kernel32.lib user32.lib gdi32.lib
# Uncomment the value below for working on a 64-bit Windows system
# PLATFORM = WIN64
PLATFORM = WIN32
!if "$(PLATFORM)" == "WIN32"
LIBFILE = wstp32i4m.lib
!else
LIBFILE = wstp64i4m.lib
!endif
addtwo.exe : addtwo.obj addtwotm.obj
LINK addtwo.obj addtwotm.obj $(LIBFILE) /OUT:addtwo.exe @<<
$(LFLAGS)
<<
addtwo.obj : addtwo.c
CL @<< addtwo.c
$(CFLAGS)
<<
addtwotm.obj : addtwotm.c
CL @<< addtwotm.c
$(CFLAGS)
<<
# Need to call wsprep to preprocess WSTP template
addtwotm.c : addtwo.tm
wsprep addtwo.tm -o addtwotm.c
2. Save the file as addtwo.mak in the WSTPExamples directory.
3. Start a command prompt window by running Start ▶ All Programs ▶ Microsoft Visual Studio 2010 ▶ Visual Studio Tools ▶ Visual Studio Command Prompt (2010) (On 64-bit Windows, use Start ▶ All Programs ▶ Microsoft Visual Studio 2010 ▶ Visual Studio Tools ▶ Visual Studio x64 Win64 Command Prompt (2010)).
4. Change to the addtwo directory.
5. Type the following command.
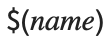
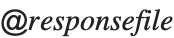
6. Using a text editor, create a file containing the following text.
# factor.mak a makefile for building the factor.exe example program
# This makefile builds a console program
CFLAGS = /nologo /c /MLd /W3 /Z7 /Od /DWIN32 /D_DEBUG /D_CONSOLE
LFLAGS = /DEBUG /PDB:NONE /NOLOGO /SUBSYSTEM:console /INCREMENTAL:no kernel32.lib user32.lib
# Uncomment the value below for working on a 64-bit Windows system
# PLATFORM = WIN64
PLATFORM = WIN32
!if "$(PLATFORM)" == "WIN32"
LIBFILE = wstp32i4m.lib
!else
LIBFILE = wstp64i4m.lib
!endif
factor.exe : factor.obj
LINK factor.obj $(LIBFILE) /OUT:factor.exe @<<
$(LFLAGS)
<<
factor.obj : factor.c
CL @<< factor.c
$(CFLAGS)
<<
7. Save the file as factor.mak in the WSTPExamples directory.
8. Start a command prompt window by running Start ▶ All Programs ▶ Microsoft Visual Studio 2010 ▶ Visual Studio Tools ▶ Visual Studio Command Prompt (2010) (On 64-bit Windows, use Start ▶ All Programs ▶ Microsoft Visual Studio 2010 ▶ Visual Studio Tools ▶ Visual Studio x64 Win64 Command Prompt (2010)).
9. Change to the factor directory.
10. Type the following two commands.
Using the Integrated Development Environment Visual Studio 2012
Steps Common to All Projects
1. Copy wstp.h from the WSTP Developer Kit to the Microsoft Visual Studio 2012 Include directory.
Developer Kit path 32-bit Windows: C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows\CompilerAdditions\wstp.h
Visual Studio 2012 Include directory 32-bit Windows: C:\Program Files\Microsoft Visual Studio 11\VC\Include
Developer Kit path 64-bit Windows: C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows-x86-64\CompilerAdditions\wstp.h
Visual Studio 2012 Include directory 64-bit Windows: C:\Program Files (x86)\Microsoft Visual Studio 11\VC\Include
2. Copy the .lib files to the Microsoft Visual Studio Lib directory.
Copy wstp32i4m.lib from: C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows\CompilerAdditions\*.lib to: C:\Program Files\Microsoft Visual Studio 11\VC\Lib
Copy wstp64i4m.lib from: C:\Program Files\Wolfram Research\Mathematica\11.0\AddOns\WSTP\DeveloperKit\Windows-x86-64\CompilerAdditions\*.lib to: C:\Program Files (x86)\Microsoft Visual Studio 11\VC\Lib\AMD64
3. Copy wsprep.exe.
from: C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows\CompilerAdditions\wsprep.exe to: C:\Program Files\Microsoft Visual Studio 11\VC\bin
from: C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows-x86-64\CompilerAdditions\wsprep.exe to: C:\Program Files (x86)\Microsoft Visual Studio 11\VC\bin\amd64
Creating a Project for addtwo.exe
1. Start Microsoft Visual Studio 2012.
2. Click File ▶ New ▶ Project.
The New Project dialog box appears.
3. Select Installed ▶ Templates ▶ Visual C++ and click the Win32 Project icon. (In Visual Studio 2012 Update 3, there is a bug that prevents Win32 Projects from correctly working with custom build tools and custom build steps as described below. If you encounter problems using the Win32 Project, instead use the Win32 Console Application project icon.)
4. In the Location text field, type:
C:\users\<username>\documents\visual studio 2012\Projects
In the Name text field, type addtwo.
The Win32 Application Wizard dialog box appears.
5. Click Application Settings. Under the Additional options set, click the Empty Project text box.
6. Uncheck the Security Development Lifecycle checks checkbox. Click Finish.
7. To build a 64-bit version of addtwo, click the Win32 drop-down menu and select Configuration Manager.
The Configuration Manager dialog appears.
Under Active solution platform, select the drop-down menu and click New.
The New Solution Platform dialog appears.
Set the New Platform: drop-down menu to x64 and the Copy settings from: drop-down to Win32.
Click OK/Close to close the dialog windows.
Click the Win32 drop-down menu and select x64.
8. Select the addtwo project in the Solution Explorer by clicking once. From the Project menu, select Project ▶ Add Existing Item.
The Add Existing Item dialog box appears.
9. Use the file chooser panes to browse to the following directory:
C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows\WSTPExamples
C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows-x86-64\WSTPExamples
10. In the File name: text box, enter "addtwo.c" "addtwo.tm" separated by spaces. Click Add.
11. A prompt box might appear asking if you want to create a "New Rule" for building .tm files. Click No.
12. In the Solution Explorer, drag the addtwo.tm file into the Source Files folder.
13. Select the addtwo project in the Solution Explorer by clicking once. From the Project menu, select Project ▶ Add New Item.
The Add New Item dialog box appears.
In the Location text field, add:
C:\Users\<username>\documents\visual studio 2012\Projects\addtwo
In the Name: text field, type addtwotm.c.
Close the addtwotm.c file editor that opens in the file editors pane.
14. Right-click the addtwo project in the Solution Explorer and select Properties.
15. Click the Expand Tree button next to Configuration Properties.
16. Click the Expand Tree button next to Linker.
18. In the Additional Dependencies text field, enter wstp32i4m.lib.
For 64-bit Windows, enter wstp64i4m.lib.
19. Click addtwo.tm in the Solution Explorer. From the Project menu, select Project ▶ Properties.
The addtwo.tm Property Pages dialog box appears.
20. Click the Expand Tree button next to Configuration Properties.
22. Change the Item Type drop-down to Custom Build Tool.
23. Click the Expand Tree button next to Custom Build Tool.
25. In the rightmost pane, click the empty box across from the Command Line. In this text box, type (include the quotes): "$(VCInstallDir)\bin\wsprep.exe" "%(FullPath)" -o "$(ProjectDir)..\addtwotm.c".
For 64-bit Windows, use: "$(VCInstallDir)\bin\amd64\wsprep.exe" "%(FullPath)" -o "$(ProjectDir)..\addtwotm.c".
26. In the text field across from the Outputs text, type: ..\addtwotm.c.
27. Right-click the addtwo project in the SolutionExplorer and select Properties.
28. Click the Expand Tree button next to Configuration Properties.
30. Click the Project Defaults expand tree button.
31. Set the drop-down menu opposite Character Set to Not Set.
32. From the Build menu, select Build ▶ Build Solution. If you experience a linker error that looks like the following and you had to switch to the Win32 Console Application project setting as mentioned earlier:
1>------ Build started: Project: addtwo, Configuration: Debug x64 ------
1> addtwo.c
1>MSVCRTD.lib(crtexe.obj) : error LNK2019: unresolved external symbol main referenced in function __tmainCRTStartup
1>c:\users\steve\documents\visual studio 2012\Projects\addtwo\x64\Debug\addtwo.exe : fatal error LNK1120: 1 unresolved externals
========== Build: 0 succeeded, 1 failed, 0 up-to-date, 0 skipped ==========
You must edit the file addtwo.c and change line 25 from
#if WINDOWS_WSTP
#if 0 && WINDOWS_WSTP
Save addtwo.c and run Build ▶ Build Solution again.
33. The addtwo.exe binary is now in:
C:\users\<username>\documents\visual studio 2012\Projects\addtwo\addtwo\Debug
C:\users\<username>\documents\visual studio 2012\Projects\addtwo\addtwo\x64\Debug
Creating a Project for factor.exe
1. Start Microsoft Visual Studio 2012.
2. Click File ▶ New ▶ Project.
The New Project dialog box appears.
3. Select Installed ▶ Templates ▶ Visual C++ and click the Win32 Console Application icon.
4. In the Location text field, type:
C:\users\<username>\documents\visual studio 2012\Projects
In the Name text field, type factor.
The Win32 Application Wizard dialog box appears.
5. Click Application Settings. Under the Additional options set, click the Empty Project text box.
6. Uncheck the Security Development Lifecycle checks checkbox. Click Finish.
7. To build a 64-bit version of factor, click the Win32 drop-down menu and select Configuration.
The Configuration Manager dialog appears.
Under Platform, select the drop-down menu and click New.
The New Project Platform dialog appears.
Set the New Platform: drop-down menu to x64 and the Copy settings from: drop-down to Win32.
Click OK to close the dialog windows.
Click the Win32 drop-down menu and select x64.
8. Select the factor project in the Solution Explorer by clicking once. From the Project menu, select Project ▶ Add Existing Item.
The Add Existing Item dialog box appears.
9. From the Look in drop-down menu, select the following directory:
C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows\WSTPExamples
C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows-x86-64\WSTPExamples
10. In the File name: text box, enter factor.c.
11. Right-click the factor project in the Solution Explorer and select Properties.
12. Click the Expand Tree button next to Configuration Properties.
13. Click the Expand Tree button next to Linker.
15. In the Additional Dependencies text field, enter wstp32i4m.lib.
For 64-bit Windows, enter wstp64i4m.lib.
16. From the Build menu, select Build ▶ Build Solution.
17. The factor.exe binary is now in:
C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows\WSTPExamples\Debug
C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows-x86-64\WSTPExamples\Debug
Using the Integrated Development Environment Visual Studio 2010
Steps Common to All Projects
1. Copy wstp.h from the WSTP Developer Kit to the Microsoft Visual Studio 2010 Include directory.
Developer Kit path 32-bit Windows: C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows\CompilerAdditions\wstp.h
Visual Studio 2010 Include directory 32-bit Windows: C:\Program Files\Microsoft Visual Studio 10\VC\Include
Developer Kit path 64-bit Windows: C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows-x86-64\CompilerAdditions\wstp.h
Visual Studio 2010 Include directory 64-bit Windows: C:\Program Files (x86)\Microsoft Visual Studio 10\VC\Include
2. Copy the .lib files to the Microsoft Visual Studio Lib directory.
Copy wstp32i4m.lib from: C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows\CompilerAdditions\ to: C:\Program Files\Microsoft Visual Studio 10\VC\Lib
Copy wstp64i4m.lib from: C:\Program Files (x86)\Wolfram Research\Mathematica\11.0\AddOns\WSTP\DeveloperKit\Windows-x86-64\CompilerAdditions\ to: C:\Program Files (x86)\Microsoft Visual Studio 10\VC\Lib\amd64
3. Copy wsprep.exe.
from: C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows\CompilerAdditions\wsprep.exe to: C:\Program Files\Microsoft Visual Studio 10\VC\bin
from: C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows-x86-64\CompilerAdditions\wsprep.exe to: C:\Program Files (x86)\Microsoft Visual Studio 10\VC\bin\amd64
Creating a Project for addtwo.exe
1. Start Microsoft Visual Studio 2010.
2. Click File ▶ New ▶ Project.
The New Project dialog box appears.
3. Under Installed Templates, select Visual C++ ▶ Win32 and click the Win32 Project icon.
4. In the Location text field, type:
C:\users\<username>\documents\visual studio 2010\Projects
In the Name text field, type addtwo.
The Win32 Application Wizard dialog box appears.
5. Click Application Settings. Under the Additional options set, click the Empty Project text box. Click Finish.
6. To build a 64-bit version of addtwo, click the Win32 drop-down menu and select Configuration.
The Configuration Manager dialog appears.
Under Platform, select the drop-down menu and click New.
The New Project Platform dialog appears.
Set the New Platform: drop-down menu to x64 and the Copy settings from: drop-down to Win32.
Click OK to close the dialog windows.
Click the Win32 drop-down menu and select x64.
7. Select the addtwo project in the Solution Explorer by clicking once. From the Project menu, select Project ▶ Add Existing Item.
The Add Existing Item dialog box appears.
8. Use the file chooser panes to browse to the following directory:
C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows\WSTPExamples
C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows-x86-64\WSTPExamples
9. In the File name: text box, enter "addtwo.c" "addtwo.tm" separated by spaces. Click Add.
10. A prompt box might appear asking if you want to create a "New Rule" for building .tm files. Click No.
11. In the Solution Explorer, drag the addtwo.tm file into the Source Files folder.
12. Select the addtwo project in the Solution Explorer by clicking once. From the Project menu, select Project ▶ Add New Item.
The Add New Item dialog box appears.
In the Location text field, add:
C:\Users\<username>\documents\visual studio 2010\Projects\addtwo
In the Name: text field, type addtwotm.c.
Close the addtwotm.c file editor that opens in the file editors pane.
13. Right-click the addtwo project in the Solution Explorer and select Properties.
14. Click the Expand Tree button next to Configuration Properties.
15. Click the Expand Tree button next to Linker.
17. In the Additional Dependencies text field, enter wstp32i4m.lib.
For 64-bit Windows, enter wstp64i4m.lib.
18. Click addtwo.tm in the Solution Explorer. From the Project menu, select Project ▶ Properties.
The addtwo.tm Property Pages dialog box appears.
19. Click the Expand Tree button next to Configuration Properties.
20. Click the Expand Tree button next to Custom Build Step.
22. In the rightmost pane, click the empty box across from the Command Line. In this text box, type (include the quotes): "$(VCInstallDir)\bin\wsprep.exe" "%(FullPath)" -o "$(ProjectDir)..\addtwotm.c".
For 64-bit Windows, use: "$(VCInstallDir)\bin\amd64\wsprep.exe" "%(FullPath)" -o "$(ProjectDir)..\addtwotm.c".
23. In the text field across from the Outputs text, type: ..\addtwotm.c.
24. Right-click the addtwo project in the SolutionExplorer and select Properties.
25. Click the Expand Tree button next to Configuration Properties.
27. Click the Project Defaults expand tree button.
28. Set the drop-down menu opposite Character Set to Not Set.
29. From the Build menu, select Build ▶ Build Solution.
30. After the project builds, Microsoft Visual Studio 2010 will display a dialog box informing you that the file addtwotm.c has changed and asking you if you would like to reload the file. Click Yes.
31. The addtwo.exe binary is now in:
C:\users\<username>\documents\visual studio 2010\Projects\addtwo\addtwo\Debug
C:\users\<username>\documents\visual studio 2010\Projects\addtwo\addtwo\x64\Debug
Creating a Project for factor.exe
1. Start Microsoft Visual Studio 2010.
2. Click File ▶ New ▶ Project.
The New Project dialog box appears.
3. In the Installed Templates pane, click the tree expand icon next to Visual C++. Select Win32. In the Templates pane, click the Win32 Console Application icon.
4. In the Location text field, type:
C:\users\<username>\documents\visual studio 2010\Projects
In the Name text field, type factor.
The Win32 Application Wizard dialog box appears.
5. Click Application Settings. Under the Additional options set, click the Empty Project text box. Click Finish.
6. To build a 64-bit version of addtwo, click the Win32 drop-down menu and select Configuration.
The Configuration Manager dialog appears.
Under Platform, select the drop-down menu and click New.
The New Project Platform dialog appears.
Set the New Platform: drop-down menu to x64 and the Copy settings from: drop-down to Win32.
Click OK to close the dialog windows.
Click the Win32 drop-down menu and select x64.
7. Select the factor project in the Solution Explorer by clicking once. From the Project menu, select Project ▶ Add Existing Item.
The Add Existing Item dialog box appears.
8. From the Look in drop-down menu, select the following directory:
C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows\WSTPExamples
C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows-x86-64\WSTPExamples
9. In the File name: text box, enter factor.c.
10. Right-click the factor project in the Solution Explorer and select Properties.
11. Click the Expand Tree button next to Configuration Properties.
12. Click the Expand Tree button next to Linker.
14. In the Additional Dependencies text field, enter wstp32i4m.lib.
15. From the Build menu, select Build ▶ Build Solution.
16. The factor.exe binary is now in:
C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows\WSTPExamples\Debug
C:\Program Files\Wolfram Research\Mathematica\11.0\SystemFiles\Links\WSTP\DeveloperKit\Windows-x86-64\WSTPExamples\Debug
Running a Prebuilt Example from the Wolfram Language Kernel
:Begin:
:Function: addtwo
:Pattern: AddTwo[i_Integer, j_Integer]
:Arguments: { i, j }
:ArgumentTypes: { Integer, Integer }
:ReturnType: Integer
:End:
:Evaluate: AddTwo::usage = "AddTwo[x, y] gives the sum of two machine integers x and y."
int addtwo( int i, int j)
{
return i+j;
}
int __stdcall WinMain( HINSTANCE hinstCurrent, HINSTANCE hinstPrevious, LPSTR lpszCmdLine, int nCmdShow)
{
char buff[512];
char FAR * buff_start = buff;
char FAR * argv[32];
char FAR * FAR * argv_end = argv + 32;
if( !WSInitializeIcon( hinstCurrent, nCmdShow)) return 1;
WSScanString( argv, &argv_end, &lpszCmdLine, &buff_start);
return WSMain( argv_end - argv, argv);
}
Invoking the Wolfram Language Kernel from within a Prebuilt Example
2. Change to the PrebuiltExamples directory.
3. Type the following commands.
The Choose a WSTP Program To Launch dialog box appears.
After a moment, a prompt will appear requesting that you type an integer.
5. Type an integer with fewer than 10 digits and press Enter. (The other factor examples relax the restriction on the size of integer you may type in.)
The prime factors returned by the Wolfram Language are printed, and factor closes its link with the Wolfram Language.
Supported Link Protocols
- Check that the WSTP system additions are located someplace where the Windows dynamic link library search mechanism can find them.
- Make sure that the WSTP system additions you are using are from the latest Developer Kit. Two programs that are using runtime components from different revisions of WSTP may not work, or if they do work may be slower than using the latest components.
- Turn off compiler optimization until your program is working. This makes compiling faster, debugging easier, and besides, the optimizer may be broken and the cause of some problems. (Optimized code uses the stack and registers differently than unoptimized code in such a way that may expose or mask a bug in your code. For example, the common mistake of returning a pointer to a local variable may or may not cause a problem depending on stack and register use.)
- Check the return values from the WSTP library functions or call WSError() at key points in your program. WSTP will often be able to tell you what has gone wrong. (If you do not assign the return value to a variable, you can still check the return value of WSTP functions using your debugger's register window. The 32-bit library returns its results in register EAX.)
- While developing your program, place the debug version wstp32i4.dll in the same folder. This library will do more extensive error checking and log information that may be useful.
- The files wstp.h, wsprep.exe, wstp32i4.dll, and the .lib import libraries are a matched set. If you have used an earlier release of WSTP, or a different interface of WSTP, you should take care that you do not mix components when building your application.
- The network control panel must show that TCP/IP is installed before you can use LinkProtocol->"TCPIP" or LinkProtocol->"TCP". Try typing telnet at a command prompt. Telnet will not function without TCP/IP installed.