ExternalEvaluate["sys","cmd"]
evaluates the command cmd in the external evaluator sys, returning an expression corresponding to the output.
ExternalEvaluate[{"sys",opts},"cmd"]
uses the options opts for the external evaluator.
ExternalEvaluate[assoc,"cmd"]
evaluates cmd using the external evaluator specified by assoc.
ExternalEvaluate[session,"cmd"]
evaluates cmd in the specified running ExternalSessionObject.
ExternalEvaluate[sys"type",…]
returns output converted to the specified type.
ExternalEvaluate[spec,obj]
evaluates the content of the specified ExternalObject, ExternalOperation, File, URL or CloudObject.
ExternalEvaluate[spec,assoc]
evaluates the command specified by assoc.
ExternalEvaluate[spec,{cmd1,cmd2,…}]
evaluates the list of commands cmdi.
ExternalEvaluate[DatabaseReference[ref],"cmd"]
evaluates cmd using the database specified by ref.
ExternalEvaluate[spec]
represents an operator form of ExternalEvaluate that can be applied to a command or object.
Details
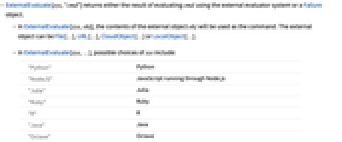
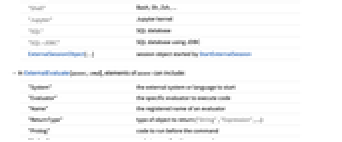
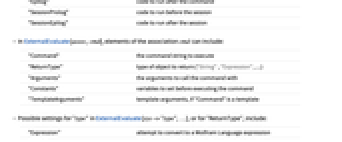
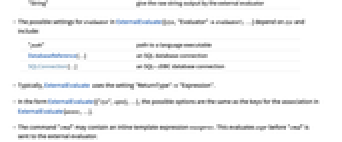
- ExternalEvaluate[sys,"cmd"] returns either the result of evaluating cmd using the external evaluator system or a Failure object.
- In ExternalEvaluate[sys,obj], the contents of the external object obj will be used as the command. The external object can be ExternalObject[…], ExternalOperation[…], File[…], URL[…], CloudObject[…] or LocalObject[…].
- In ExternalEvaluate[sys,…], possible choices of sys include:
-
"Python" Python "NodeJS" JavaScript running through Node.js "Julia" Julia "Ruby" Ruby "R" R "Java" Java "Octave" Octave "Shell" Bash, Sh, Zsh, ... "Jupyter" Jupyter kernel "SQL" SQL database "SQL-JDBC" SQL database using JDBC ExternalSessionObject[…] session object started by StartExternalSession - In ExternalEvaluate[assoc,cmd], elements of assoc can include:
-
"System" the external system or language to start "Evaluator" the specific evaluator to execute code "Name" the registered name of an evaluator "ReturnType" type of object to return ("String", "Expression", ...) "Prolog" code to run before the command "Epilog" code to run after the command "SessionProlog" code to run before the session "SessionEpilog" code to run after the session - In ExternalEvaluate[spec,cmd], elements of the association cmd can include:
-
"Command" the command to execute "ReturnType" type of object to return ("String", "Expression", ...) "Arguments" the arguments to call the command with "Constants" variables to set before executing the command "TemplateArguments" template arguments, if "Command" is a template - Possible settings for obj in ExternalEvaluate[spec,obj], or for "Command" include:
-
"code" the string to execute ExternalOperation[…] the external operation to execute ExternalObject[…] the external object to retrieve ExternalFunction[…] the external function to retrieve File[…] the file contents to execute URL[…] the contents given by the URL to execute CloudObject[…] the command specified by the cloud object LocalObject[…] the command specified by the local object - Possible settings for "type" in ExternalEvaluate[sys->"type",…], or for "ReturnType", include:
-
"Expression" attempt to convert to a Wolfram Language expression "String" give the raw string output by the external evaluator "ExternalObject" return the result as ExternalObject - The possible settings for evaluator in ExternalEvaluate[{sys,"Evaluator"evaluator},…] depend on sys and include:
-
"path" path to a language executable DatabaseReference[…] an SQL database connection SQLConnection[…] an SQL-JDBC database connection - The default "ReturnType" varies from system to system; typically it is "Expression".
- In the form ExternalEvaluate[{"sys",opts},…], the possible options are the same as the keys for the association in ExternalEvaluate[assoc,…].
- The command "cmd" may contain an inline template expression <*expr*>. This evaluates expr before "cmd" is sent to the external evaluator.
- ExternalEvaluate[sys,cmd] launches the external evaluator, evaluates cmd, then exits the external session.
- ExternalEvaluate[session,cmd] sends the command to a running session and does not exit the session.
- When sys is specified as a string, the session options used are searched first for user-specified options specified using RegisterExternalEvaluator, then from built-in session options included with the system or automatically discovered.
- FindExternalEvaluators gives a dataset of evaluator systems that can be used.
- For most external evaluators, individual write operations to standard output are immediately printed to the notebook or terminal.
Examples
open allclose allBasic Examples (10)
Evaluate a simple arithmetic expression in Python:
Evaluate a basic math function in JavaScript using Node.js:
Import a library in Python and use a function:
Evaluate multiple lines of code in a Python session:
Use the File wrapper to execute code contained in a file:
Deploy code using CloudDeploy and then run the code directly from a CloudObject:
Use a URL wrapper to directly run code hosted online:
A Julia dictionary is returned as an Association:
Evaluate a function by name using a single Wolfram Language expression as the argument:
Evaluate a query in a database and return the result:
The same data can be returned as Tabular:
Use > at the beginning of a line to start an external code cell evaluated with ExternalEvaluate:
Evaluate code in a Python provisioned environment:
Scope (36)
Basic Examples (4)
Compute powers of 10 using a range of numbers in Python:
Returning the result as a Python dict gives an Association:
Many types, like datetime, are natively mapped to Wolfram Language expressions:
Add two numbers in Python using an inline TemplateExpression:
Use ExternalOperation to compose complex operations:
Session Options (13)
"ReturnType" (4)
For most systems, the default return type is "Expression":
Numbers, strings, lists and associations are automatically imported for the "Expression" return type:
The return type of "String" returns a string of the result in the external language:
When using a database, the default return type is "Dataset":
"ReturnType" can be used to return data in a different form:
"Evaluator" (3)
Evaluate code using a specified "Evaluator":
When using a File with the "SQL" evaluator, the evaluator can be a path to an SQLite file or a DatabaseReference specification:
An Association can be used to evaluate code in a Python provisioned environment:
"Name" (1)
"SessionProlog" (2)
Command Options (19)
"Command" (7)
When a string is provided, the command is directly executed:
The above is equivalent to writing the command using this form:
Use a File wrapper to run the code from a file:
The above is equivalent to writing the command using this form:
Use a URL wrapper to directly run code hosted online:
The above is equivalent to writing the command using this form:
Put code in a CloudObject:
Evaluate directly from the cloud:
The above is equivalent to writing the command using this form:
ExternalOperation can be used in "Command":
If needed, arguments can be used to call the operation:
ExternalFunction can be used in "Command":
Create an ExternalObject:
Call the object using "Command" and "Arguments":
When using only "Command", the object will be casted to the default "ReturnType":
"ReturnType" (1)
"Arguments" (4)
Use "Arguments" to call the command with arguments:
For a single argument, you do not need to use a list:
If you need to pass a list as first argument, you need to wrap it with an extra list explicitly:
You can define a function inside "Command" and directly call it with "Arguments":
The same result can be achieved by using a Rule:
An alternative method is to define an ExternalFunction:
ExternalOperation can be used in "Arguments":
Create an ExternalObject:
"Constants" (3)
Use "Constants" to permanently set global variables before the command runs:
ExternalOperation can be used in "Constants":
ExternalFunction can be used in "Constants":
"TemplateArguments" (4)
When running a command, you can inline a TemplateExpression:
You can explicitly fill TemplateSlot using "TemplateArguments":
If you need to pass a list as first argument, you need to wrap it with an extra list explicitly:
You can name template slots and use an Association to pass named arguments to the template:
ExternalOperation can be used in "TemplateArguments":
ExternalFunction can be used in "TemplateArguments":
Properties & Relations (4)
When an evaluation fails, a Failure object is returned:
The type of exception thrown is accessible inside the failure object:
External language cells implicitly call StartExternalSession:
A new session has been started:
By default, all cells of a given system use that session:
End the session so that subsequent evaluations start in a new session:
The same session is used when running a list of commands:
Separate sessions are used in a list of separate ExternalEvaluate calls:
The "String" and "Expression" return types can be related using import and export:
Use ImportString with "PythonExpression" to convert a Python string to an expression:
Conversely, use ExportString to convert an expression to its Python string:
Possible Issues (1)
External language cells implicitly call StartExternalSession and use a single session:
Unless given an ExternalSessionObject, each ExternalEvaluate call uses a separate session:
For persistent evaluation, use StartExternalSession:
Text
Wolfram Research (2017), ExternalEvaluate, Wolfram Language function, https://reference.wolfram.com/language/ref/ExternalEvaluate.html (updated 2024).
CMS
Wolfram Language. 2017. "ExternalEvaluate." Wolfram Language & System Documentation Center. Wolfram Research. Last Modified 2024. https://reference.wolfram.com/language/ref/ExternalEvaluate.html.
APA
Wolfram Language. (2017). ExternalEvaluate. Wolfram Language & System Documentation Center. Retrieved from https://reference.wolfram.com/language/ref/ExternalEvaluate.html